This is a Simple Wallpaper Manager that allows you to select image files from a location on your computer and build saveable lists of local wallpaper image files that can then be loaded in to the application to save browse time. Automatic wallpaper cycle allows you to configure automatic wallpaper change every hour, daily, weekly, or monthly.
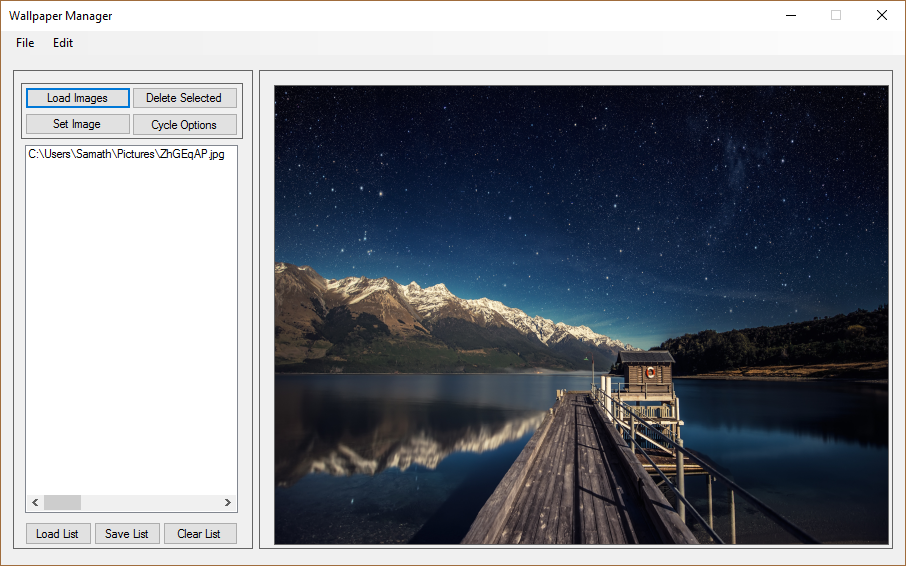
Code:
Public Class FormMain
Dim w As IO.StreamWriter 'saving list
Dim r As IO.StreamReader 'loading list
Private Sub Button1_Click(sender As System.Object, e As System.EventArgs) Handles btnLoad.Click
'Select our wallpaper image files for list and preview images.
If OpenFileDialog1.ShowDialog() = DialogResult.OK Then
PictureBox1.Load(OpenFileDialog1.FileName)
End If
Dim file As String
'Build wallpaper list.
For Each file In OpenFileDialog1.FileNames
ListBox1.Items.Add(file)
Next
End Sub
Private Sub ListBox1_SelectedIndexChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ListBox1.SelectedIndexChanged
'Manually cycle through list previews.
PictureBox1.ImageLocation = (Me.ListBox1.SelectedItem)
End Sub
Private Sub btnDelete_Click(sender As System.Object, e As System.EventArgs) Handles btnDelete.Click
'Remove selected image files from list.
ListBox1.Items.Remove(ListBox1.SelectedItem)
End Sub
Private Sub btnSet_Click(sender As System.Object, e As System.EventArgs) Handles btnSet.Click
'Open wallpaper setting dialog.
Dim thirdform As New FormSelect
thirdform.Show()
End Sub
Private Sub btnSave_Click(sender As System.Object, e As System.EventArgs) Handles btnSave.Click
'Save current wallpaper list.
Dim i As Integer
If SaveFileDialog1.ShowDialog() = Windows.Forms.DialogResult.OK Then
w = New IO.StreamWriter(SaveFileDialog1.FileName)
For i = 0 To ListBox1.Items.Count - 1
w.WriteLine(ListBox1.Items.Item(i))
Next
w.Close()
MsgBox("List saved to" + SaveFileDialog1.FileName)
End If
End Sub
Private Sub Button1_Click_1(sender As System.Object, e As System.EventArgs) Handles btnLoadList.Click
'Load wallpaper list.
If OpenFileDialog2.ShowDialog() = DialogResult.OK Then
ListBox1.Items.Clear()
r = New IO.StreamReader(OpenFileDialog2.FileName)
While (r.Peek() > -1)
ListBox1.Items.Add(r.ReadLine)
End While
r.Close()
End If
End Sub
Private Sub btnClear_Click(sender As System.Object, e As System.EventArgs) Handles btnClear.Click
'Clear current wallpaper list.
ListBox1.Items.Clear()
End Sub
Private Sub btnOpt_Click(sender As System.Object, e As System.EventArgs) Handles btnOpt.Click
Dim fourthform As New FormOpt
fourthform.Show()
Me.Hide()
End Sub
'Start Menu Strip Procedures.
Private Sub BrowseToolStripMenuItem_Click(sender As System.Object, e As System.EventArgs) Handles BrowseToolStripMenuItem.Click
If OpenFileDialog1.ShowDialog() = DialogResult.OK Then
PictureBox1.Load(OpenFileDialog1.FileName)
End If
Dim file As String
For Each file In OpenFileDialog1.FileNames
ListBox1.Items.Add(file)
Next
End Sub
Private Sub LoadListToolStripMenuItem_Click(sender As System.Object, e As System.EventArgs) Handles LoadListToolStripMenuItem.Click
If OpenFileDialog2.ShowDialog() = DialogResult.OK Then
ListBox1.Items.Clear()
r = New IO.StreamReader(OpenFileDialog2.FileName)
While (r.Peek() > -1)
ListBox1.Items.Add(r.ReadLine)
End While
r.Close()
End If
End Sub
Private Sub SetSelectedWallpaperToolStripMenuItem_Click(sender As System.Object, e As System.EventArgs) Handles SetSelectedWallpaperToolStripMenuItem.Click
Dim thirdform As New FormSelect
thirdform.Show()
End Sub
Private Sub SaveListToolStripMenuItem_Click(sender As System.Object, e As System.EventArgs) Handles SaveListToolStripMenuItem.Click
Dim i As Integer
If SaveFileDialog1.ShowDialog() = Windows.Forms.DialogResult.OK Then
w = New IO.StreamWriter(SaveFileDialog1.FileName)
For i = 0 To ListBox1.Items.Count - 1
w.WriteLine(ListBox1.Items.Item(i))
Next
w.Close()
MsgBox("List saved to" + SaveFileDialog1.FileName)
End If
End Sub
Private Sub DeleteSelectedToolStripMenuItem_Click(sender As System.Object, e As System.EventArgs) Handles DeleteSelectedToolStripMenuItem.Click
ListBox1.Items.Remove(ListBox1.SelectedItem)
End Sub
End Class
Imports Microsoft.Win32
Imports System.Environment
Imports System.Drawing.Imaging
Imports System.ComponentModel
Imports System.Runtime.InteropServices
Public Class FormSelect
'Declarations for wallpaper set procedure.
Private Declare Function SystemParametersInfo Lib "user32" Alias "SystemParametersInfoA" (ByVal uAction As Integer, ByVal uParam As Integer, ByVal lpvParam As String, ByVal fuWinIni As Integer) As Integer
Private Const SETDESKWALLPAPER As Integer = &H14
Private Const UPDATEINIFILE = &H1
Private Const SPIF_SENDWININICHANGE = &H2
'End set procedure Declarations.
Private Sub Button1_Click(sender As System.Object, e As System.EventArgs) Handles Button1.Click
'Assign list data from list generated in FormMain.
Dim listdata As String
listdata = FormMain.PictureBox1.ImageLocation
'Declare registry section to be edited for wallpaper size mode.
Dim key As RegistryKey = Registry.CurrentUser.OpenSubKey("Control Panel\Desktop", True)
'Check to verify a size mode has been selected. If false, alert user and exit code sub.
'If true, edit registry keys for selected size mode and run wallpaper set procedure.
If CheckBox1.CheckState = CheckState.Unchecked And
CheckBox2.CheckState = CheckState.Unchecked And
CheckBox3.CheckState = CheckState.Unchecked Then
MsgBox("Please select a size option.")
Exit Sub
ElseIf CheckBox1.CheckState = CheckState.Checked Then
key.SetValue("WallpaperStyle", "2")
key.SetValue("TileWallpaper", "0")
SystemParametersInfo(SETDESKWALLPAPER, 0, listdata, UPDATEINIFILE)
ElseIf CheckBox2.CheckState = CheckState.Checked Then
key.SetValue("WallpaperStyle", "0")
key.SetValue("TileWallpaper", "0")
SystemParametersInfo(SETDESKWALLPAPER, 0, listdata, UPDATEINIFILE)
ElseIf CheckBox3.CheckState = CheckState.Checked Then
key.SetValue("WallpaperStyle", "0")
key.SetValue("TileWallpaper", "1")
SystemParametersInfo(SETDESKWALLPAPER, 0, listdata, UPDATEINIFILE)
End If
Me.Close()
End Sub
Private Sub Button2_Click(sender As System.Object, e As System.EventArgs) Handles Button2.Click
Me.Close()
End Sub
End Class