The clipboard is a software facility used for short-term data storage and/or data transfer between documents or applications, via copy and paste operations. It is most commonly a part of a GUI environment and is usually implemented as an anonymous, temporary data buffer, sometimes called the paste buffer, that can be accessed from most or all programs within the environment via defined programming interfaces.
This is a basic example of using the .Net frameworks builtin Clipboard object to copy and paste various data type's from your application.
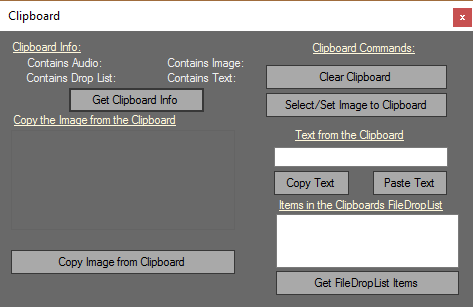
Code:
Imports System.IO
Public Class frmMain
Private Sub btnGetImage_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnGetImage.Click
Dim a As String = Nothing
Dim b As Collection = New Collection
Try
If Clipboard.ContainsImage Then
pic.Image = Clipboard.GetImage
Exit Sub
End If
If Clipboard.GetFileDropList.Count > 0 Then
For Each a In My.Computer.Clipboard.GetFileDropList
b.Add(a)
Next
Else
MessageBox.Show("There does not appear to be any image available to get from the Clipboards FileDropList.", " No Image", MessageBoxButtons.OK, MessageBoxIcon.Information)
Exit Sub
End If
If b.Count > 0 Then
a = LCase(b.Item(1).ToString)
Else
MessageBox.Show("There are not any items in the clipboard.", " Error", MessageBoxButtons.OK, MessageBoxIcon.Information)
Exit Sub
End If
If a.EndsWith(".bmp") Then
pic.Image = Image.FromFile(a.ToString)
ElseIf a.EndsWith(".gif") Then
pic.Image = Image.FromFile(a.ToString)
ElseIf a.EndsWith(".jpg") Then
pic.Image = Image.FromFile(a.ToString)
Else
MessageBox.Show("The clipboard does not contain either a Bitmap, Gif, or JPeg image to paste from!", " No Image to Paste", MessageBoxButtons.OK, MessageBoxIcon.Exclamation)
Exit Sub
End If
Catch exc As Exception
MessageBox.Show(exc.Message, " Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
End Try
End Sub
Private Sub btnGetItems_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnGetItems.Click
Dim item As String = Nothing
Try
If Clipboard.ContainsFileDropList <> 0 Then
For Each item In Clipboard.GetFileDropList
lstFileDropItems.Items.Add(item)
Next
Else
MessageBox.Show("There does not appear to be a FileDropList with any items in it!", " No FileDropList", MessageBoxButtons.OK, MessageBoxIcon.Information)
Exit Sub
End If
Catch exc As Exception
MessageBox.Show(exc.Message, " Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
End Try
End Sub
Private Sub btnSetText_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnSetText.Click
Try
If Not txtText.Text = "" Then
My.Computer.Clipboard.SetText(txtText.Text)
Else
My.Computer.Clipboard.SetText("This is just a test!")
End If
Catch exc As Exception
MessageBox.Show(exc.Message, " Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
End Try
End Sub
Private Sub btnGetText_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnGetText.Click
Try
If My.Computer.Clipboard.ContainsText Then
txtText.Text = My.Computer.Clipboard.GetText.ToString
Else
MessageBox.Show("The clipboard does not contain any text to paste to the textbox control!", " No TEXT in Clipboard", MessageBoxButtons.OK, MessageBoxIcon.Information)
End If
Catch exc As Exception
MessageBox.Show(exc.Message, " Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
End Try
End Sub
Private Sub btnLoadInfo_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnLoadInfo.Click
Try
lblContainsAudio.Text = "Contains Audio: " & My.Computer.Clipboard.ContainsAudio.ToString
lblFileDropList.Text = "Contains Drop List: " & My.Computer.Clipboard.ContainsFileDropList.ToString
lblContainsImage.Text = "Contains Image: " & My.Computer.Clipboard.ContainsImage.ToString
lblContainsText.Text = "Contains Text: " & My.Computer.Clipboard.ContainsText.ToString
Catch exc As Exception
MessageBox.Show(exc.Message, " Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
End Try
End Sub
Private Sub btnClear_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnClear.Click
Try
DialogResult = _
MessageBox.Show("Are you sure you want to clear?", _
" Clear the clipboard?", MessageBoxButtons.YesNo, _
MessageBoxIcon.None)
If DialogResult = Windows.Forms.DialogResult.Yes Then
My.Computer.Clipboard.Clear()
End If
Catch exc As Exception
MessageBox.Show(exc.Message, " Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
End Try
End Sub
Private Sub btnSetSelectImage_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnSetSelectImage.Click
Try
Dim openDLG As OpenFileDialog = New OpenFileDialog
openDLG.Filter = "Image Files (*.bmp, *.gif, *.jpg)|*.bmp;*.gif;*.jpg"
DialogResult = openDLG.ShowDialog
If DialogResult = Windows.Forms.DialogResult.OK Then
Clipboard.Clear()
Clipboard.SetImage(Image.FromFile(openDLG.FileName))
If Clipboard.ContainsImage Then
MessageBox.Show("The image should now be pasted in the clipboard.", " Image Successfully Pasted!", MessageBoxButtons.OK, MessageBoxIcon.Information)
Else
MessageBox.Show("The image does not appear to have been successfully pasted to the clipboard!", " Possible Problem", MessageBoxButtons.OK, MessageBoxIcon.Exclamation)
End If
End If
Catch exc As Exception
MessageBox.Show(exc.Message, " Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
End Try
End Sub
End Class