The following table lists the freezing and boiling points of several substances.
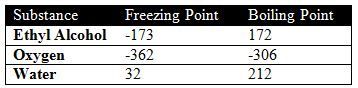
Design a class that stores a temperature in a temperature member variable and has the appropriate getter and setter functions. In addition to appropriate constructors, the class should have the following member functions:
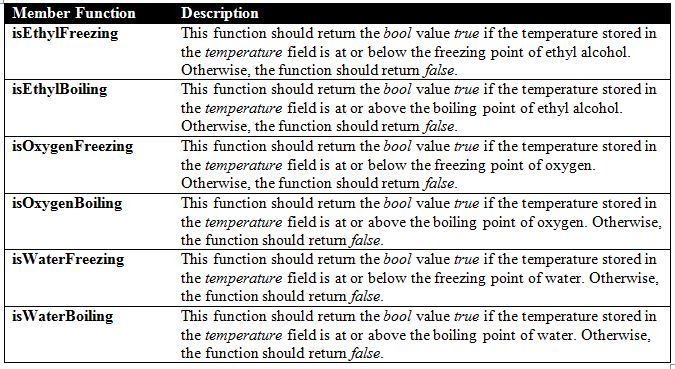
Write a program (driver.cpp) that demonstrates the class. The program should ask the user to enter different temperatures, and then display a list of the substances that will freeze at that temperature and those that will boil at that temperature.
Temperature.h
#ifndef TEMPH
#define TEMPH
#include <iostream>
#include <cstdlib>
using namespace std;
class Temperature
{
public:
Temperature(int temp);
bool isEthylFreezing();
bool isEthylBoiling();
bool isOxygenFreezing();
bool isOxygenBoiling();
bool isWaterFreezing();
bool isWaterBoiling();
void setTemperature(int temp);
int getTemperature();
private:
int temperature;
};
#endif
Temperature.cpp
#include <iostream>
#include <cstdlib>
#include "Temperature.h"
using namespace std;
Temperature::Temperature(int temp)
{
temperature = temp;
}
bool Temperature::isEthylFreezing()
{
if(temperature<=-173)
{
return true;
}
else
{
return false;
}
}
bool Temperature::isEthylBoiling()
{
if(temperature>=172)
{
return true;
}
else
{
return false;
}
}
bool Temperature::isOxygenFreezing()
{
if(temperature<=-362)
{
return true;
}
else
{
return false;
}
}
bool Temperature::isOxygenBoiling()
{
if(temperature>=-306)
{
return true;
}
else
{
return false;
}
}
bool Temperature::isWaterFreezing()
{
if(temperature<=32)
{
return true;
}
else
{
return false;
}
}
bool Temperature::isWaterBoiling()
{
if(temperature>=212)
{
return true;
}
else
{
return false;
}
}
void Temperature::setTemperature(int temp)
{
temperature = temp;
}
int Temperature::getTemperature()
{
return temperature;
}
driver.cpp
#include <cstdlib>
#include <iostream>
#include "Temperature.h"
using namespace std;
int main(int argc, char *argv[])
{
int my_temp;
cout<<"Enter a Temperature: ";
cin>>my_temp;
Temperature temp(10);
temp.setTemperature(my_temp);
if(temp.isEthylFreezing())
{
cout<<"Ethyl Alcohol will Freeze at this Temperature.\n";
}
if(temp.isOxygenFreezing())
{
cout<<"Oxygen will Freeze at this Temperature.\n";
}
if(temp.isWaterFreezing())
{
cout<<"Water will Freeze at this Temperature.\n";
}
if(temp.isEthylBoiling())
{
cout<<"Ethyl Alcohol will Boil at this Temperature.\n";
}
if(temp.isOxygenBoiling())
{
cout<<"Oxygen will Boil at this Temperature.\n";
}
if(temp.isWaterBoiling())
{
cout<<"Water will Boil at this Temperature.\n";
}
system("PAUSE");
return 0;
}