Write a Circle class that has the following member variables:
• radius: double
• pi: a double initialized with the value 3.14159
The class should have the following member function:
• Default constructor: A default constructor that sets radius to 1.0
• Constructor: Accepts the radius of the circle as argument. You are required to use exception handling techniques as discussed in class, if a user enters 0.0 for the radius, throw an exception informing the user that the radius cannot be 0.0 and allow them to enter a new value.
• setRadius: A mutator function for the radius variable
• getRadius: An accessor function for the radius variable
• getArea: Returns the area of a circle, which is calculated as area = pi* radius * radius.
• getDiameter: return the diameter of the circle, which is calculated as radius * 2.
• getCircumference: returns the circumference of the circle, which is calculated as circumference = 2 * pi * radius.
You are required to demonstrate your class in a driver program.
Deliverables: Circle.h, Circle.cpp, tester.cpp, ZeroException.h
Output:
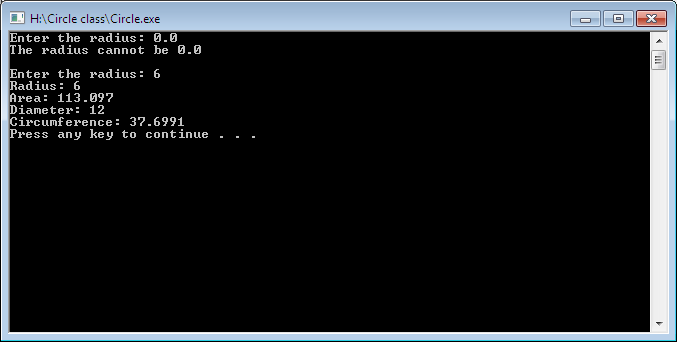
Circle.cpp
#include <iostream>
#include <cstdlib>
#include "Circle.h"
#include "ZeroException.h"
using namespace std;
Circle::Circle()
{
radius = 1.0;
}
Circle::Circle(double radi)
{
if( radi == 0 || radi == 0.0)
{
ZeroException ex;
ex.Checkradius();
}
else
{
radius = radi;
}
}
void Circle::setRadius(double r)
{
if( r == 0 || r == 0.0)
{
ZeroException ex;
ex.Checkradius();
}
else
{
radius = r;
}
}
double Circle::getRadius()
{
return radius;
}
double Circle::getArea()
{
return pi * radius * radius;
}
double Circle::getDiameter()
{
return radius * 2;
}
double Circle::getCircumference()
{
return 2 * pi * radius;
}
void ZeroException::Checkradius()
{
throw 0.0;
}
Circle.h
#ifndef CIRCLEH
#define CIRCLEH
#include <iostream>
#include <cstdlib>
using namespace std;
class Circle
{
public:
Circle();
Circle(double radi);
void setRadius(double r);
double getRadius();
double getArea();
double getDiameter();
double getCircumference();
private:
double radius;
static const double pi = 3.14159;
};
#endif
ZeroException.h
#ifndef ZeroExceptionH
#define ZeroExceptionH
#include <iostream>
#include <cstdlib>
using namespace std;
class ZeroException
{
public:
void Checkradius();
};
#endif
Tester.cpp
#include <cstdlib>
#include <iostream>
#include "Circle.h"
using namespace std;
int main(int argc, char *argv[])
{
for(;;)
{
try
{
double radius;
cout<<"Enter the radius: ";
cin>>radius;
Circle cir(radius);
cout<<"Radius: "<<cir.getRadius()<<endl;
cout<<"Area: "<<cir.getArea()<<endl;
cout<<"Diameter: "<<cir.getDiameter()<<endl;
cout<<"Circumference: "<<cir.getCircumference()<<endl;
break;
}
catch(double e)
{
cout<<"The radius cannot be 0.0"<<endl<<endl;
continue;
}
}
system("PAUSE");
return 0;
}