Roman numerals are an ancient number system but still have uses in the modern world. Roman numerals are used to represent dates, analog clock faces and for indexing. This C# code will show you how to convert a number into Roman numeral.
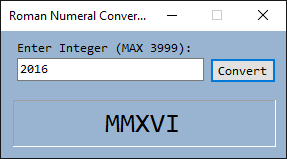
Code:
using System;
using System.Windows.Forms;
namespace IntegertoRomanNumeral
{
public partial class FormConvertor : Form
{
public FormConvertor()
{
InitializeComponent();
}
private void btnconvert_Click(object sender, EventArgs e)
{
lblroman.ResetText();
int number = Convert.ToInt32(txtroman.Text);
if (number.ToString().Trim().Length == 0)
return;
if (number >= 4000)
{
MessageBox.Show("Please enter a number that is less than 4000.",
this.Text, MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
txtroman.Focus();
return;
}
String[] roman = new String[] { "M", "CM", "D", "CD", "C", "XC", "L", "XL", "X", "IX", "V", "IV", "I" };
int[] decimals = new int[] { 1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1 };
string romanvalue = String.Empty;
for (int i = 0; i < 13; i++)
{
while (number >= decimals[i])
{
number -= decimals[i];
romanvalue += roman[i];
}
}
lblroman.Text = romanvalue;
}
}
}