This is a simple calculator written using the C# programming language. This calculator is similar to a small handheld calculator and has the standard four functions for addition, subtraction, division, and multiplication. You can use your numeric keypad to insert numbers along with the keys 'equals', 'backspace', 'delete', as well as the + - * / keys.
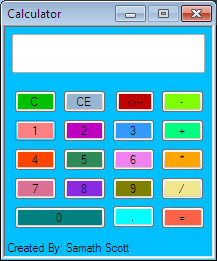
Code:
using System;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
float num1, ans;
int count;
private void btnC_Click_1(object sender, EventArgs e)
{
textBox1.Clear();
count = 0;
}
private void btnCE_Click(object sender, EventArgs e)
{
if (num1==0 && textBox1.TextLength>0)
{
num1 = 0; textBox1.Clear();
}
else if (num1 > 0 && textBox1.TextLength > 0)
{
textBox1.Clear();
}
}
private void btnback_Click(object sender, EventArgs e)
{
int lenght = textBox1.TextLength-1;
string text = textBox1.Text;
textBox1.Clear();
for (int i = 0; i < lenght; i++)
textBox1.Text = textBox1.Text + text[i];
}
private void btnminus_Click(object sender, EventArgs e)
{
if (textBox1.Text != "")
{
num1 = float.Parse(textBox1.Text);
textBox1.Clear();
textBox1.Focus();
count = 1;
}
}
private void btnone_Click(object sender, EventArgs e)
{
textBox1.Text = textBox1.Text + 1;
}
private void bttntwo_Click(object sender, EventArgs e)
{
textBox1.Text = textBox1.Text + 2;
}
private void btnthree_Click(object sender, EventArgs e)
{
textBox1.Text = textBox1.Text + 3;
}
private void btnplus_Click(object sender, EventArgs e)
{
num1 = float.Parse(textBox1.Text);
textBox1.Clear();
textBox1.Focus();
count = 2;
}
private void btnfour_Click(object sender, EventArgs e)
{
textBox1.Text = textBox1.Text + 4;
}
private void btnfive_Click(object sender, EventArgs e)
{
textBox1.Text = textBox1.Text + 5;
}
private void btnsix_Click(object sender, EventArgs e)
{
textBox1.Text = textBox1.Text + 6;
}
private void btnmultiply_Click(object sender, EventArgs e)
{
num1 = float.Parse(textBox1.Text);
textBox1.Clear();
textBox1.Focus();
count = 3;
}
private void btnseven_Click(object sender, EventArgs e)
{
textBox1.Text = textBox1.Text + 7;
}
private void btneight_Click(object sender, EventArgs e)
{
textBox1.Text = textBox1.Text + 8;
}
private void btnnine_Click(object sender, EventArgs e)
{
textBox1.Text = textBox1.Text + 9;
}
private void btndivide_Click(object sender, EventArgs e)
{
num1 = float.Parse(textBox1.Text);
textBox1.Clear();
textBox1.Focus();
count = 4;
}
private void btnzero_Click(object sender, EventArgs e)
{
textBox1.Text = textBox1.Text + 0;
}
private void btnperiod_Click(object sender, EventArgs e)
{
int c = textBox1.TextLength;
int flag = 0;
string text = textBox1.Text;
for (int i = 0; i < c; i++)
{
if (text[i].ToString() == ".")
{
flag = 1; break;
}
else
{
flag = 0;
}
}
if (flag == 0)
{
textBox1.Text = textBox1.Text + ".";
}
}
private void btnequal_Click(object sender, EventArgs e)
{
compute(count);
}
public void compute(int count)
{
switch (count)
{
case 1:
ans = num1 - float.Parse(textBox1.Text);
textBox1.Text = ans.ToString();
break;
case 2:
ans = num1 + float.Parse(textBox1.Text);
textBox1.Text = ans.ToString();
break;
case 3:
ans = num1 * float.Parse(textBox1.Text);
textBox1.Text = ans.ToString();
break;
case 4:
ans = num1 / float.Parse(textBox1.Text);
textBox1.Text = ans.ToString();
break;
default:
break;
}
}
}
}