Grade point average (GPA) is an indicator of an individual's academic achievement. It is the average of the grades attained in each course, taking course credit into consideration. This GPA calculator was written using the C# programming language; it calculates the GPA for 7 courses.
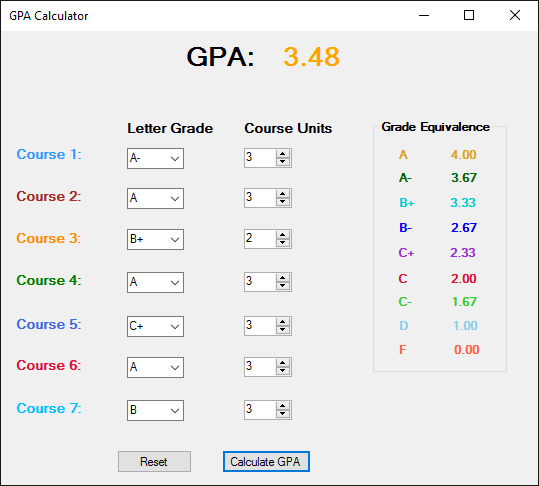
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace GPA_Calculator
{
public partial class Form1 : Form
{
String lettergrd;
double credit;
double caltimes = 0;
double totalcal = 0;
double totalcredit = 0;
double finalgpa = 0;
double A = 4.0;
double AMINUS = 3.67;
double BPLUS = 3.33;
double B = 3.0;
double BMINUS = 2.67;
double CPLUS = 2.33;
double C = 2.0;
double CMINUS = 1.67;
double D = 1.00;
double F = 0.0;
ComboBox defaultcombo = new ComboBox();
NumericUpDown defaultnumeric = new NumericUpDown();
public Form1()
{
InitializeComponent();
}
private void btncalculateGPA_Click(object sender, EventArgs e)
{
lettergrd = "";
credit = 0;
caltimes = 0;
totalcal = 0;
totalcredit = 0;
finalgpa = 0;
defaultcombo = new ComboBox();
defaultnumeric = new NumericUpDown();
for(int i = 0; i < 7; i++)
{
switch(i)
{
case 0:
defaultcombo = cmbCourse1;
defaultnumeric = numCourse1;
break;
case 1:
defaultcombo = cmbCourse2;
defaultnumeric = numCourse2;
break;
case 2:
defaultcombo = cmbCourse3;
defaultnumeric = numCourse3;
break;
case 3:
defaultcombo = cmbCourse4;
defaultnumeric = numCourse4;
break;
case 4:
defaultcombo = cmbCourse5;
defaultnumeric = numCourse5;
break;
case 5:
defaultcombo = cmbCourse6;
defaultnumeric = numCourse6;
break;
case 6:
defaultcombo = cmbCourse7;
defaultnumeric = numCourse7;
break;
}
lettergrd = defaultcombo.Text;
credit = Double.Parse(defaultnumeric.Value.ToString());
if(lettergrd != String.Empty && credit != 0)
{
switch(lettergrd)
{
case "A": caltimes = credit * A;
break;
case "A-": caltimes = credit * AMINUS;
break;
case "B+": caltimes = credit * BPLUS;
break;
case "B": caltimes = credit * B;
break;
case "B-": caltimes = credit * BMINUS;
break;
case "C+": caltimes = credit * CPLUS;
break;
case "C": caltimes = credit * C;
break;
case "C-": caltimes = credit * CMINUS;
break;
case "D": caltimes = credit * D;
break;
case "F": caltimes = credit * F;
break;
}
totalcredit = totalcredit + credit;
totalcal = totalcal + caltimes;
}
}
finalgpa = totalcal/totalcredit;
lblgpa.Text = finalgpa.ToString("#.00");
}
private void btnreset_Click(object sender, EventArgs e)
{
cmbCourse1.ResetText();
cmbCourse2.ResetText();
cmbCourse3.ResetText();
cmbCourse4.ResetText();
cmbCourse5.ResetText();
cmbCourse6.ResetText();
cmbCourse7.ResetText();
numCourse1.ResetText();
numCourse2.ResetText();
numCourse3.ResetText();
numCourse4.ResetText();
numCourse5.ResetText();
numCourse6.ResetText();
numCourse7.ResetText();
}
}
}