import java.util.Scanner;
public class Test
{
public static void main(String args[])
{
String courseCode;
int credit;
Scanner s = new Scanner(System.in);
System.out.println("Enter course code: ");
courseCode = s.nextLine();
System.out.println("Enter number of credits: ");
credit = Integer.parseInt(s.nextLine());
}
}
Review the code above to ensure that you understand how it works before proceeding.
1. Introduce a while loop so that the program will prompt the user for Course code and number of credits until the course code “END” is input.
2. Test the program with different inputs. Include one case where you input an invalid value for the number of credits. Try “six”. The program should terminate abnormally because of an exception. Please read the message that is displayed carefully and note the exception that has occurred.
3. Use a try-catch statement so that the program displays an error message instead of crashing if the user inputs an invalid value for the number of credits.
4. A valid computing course code always starts with four letters, for example “COMP”, followed by an integer value for the year the course is offered (from 1 to 3 inclusive) and ends with a 3 digit integer number. Modify the program to display an error message if an invalid computing course code is inputted.
Test your program with the following data:
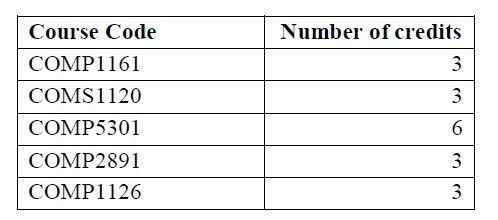
Exercise #2
1. Test your program by inputting a computing course code that is shorter than 8 digits. An exception should occur. Use a try-catch statement to prevent this from happening. The catch clause should also keep a count of the invalid cases.
2. Modify the program so that all valid computing course code information is written to a text file. {Consult your lecture slides in order to learn how this is done.}
Exercise #3
Write a class CourseCodeException that extends the Exception class. The constructor for this class should accept a string which states “Unacceptable Computing Course Code”. Modify your program to throw this exception if the course code is incorrect. Of course, your code should also catch the exception and handle it.
CourseCodeException Class:
public class CourseCodeException extends Exception
{
public CourseCodeException(String ex)
{
super(ex);
}
}
Code:
import java.io.*;
import java.util.Scanner;
import java.lang.*;
public class Test
{
public static void main(String args[]) throws IOException
{
String courseCode = "";
int credit = 0;
String temp="";
int errorCount = 0;
Scanner fileScan;
Scanner s = new Scanner(System.in);
while(!courseCode.equals("END"))
{
try
{
System.out.println("Enter course code: ");
courseCode = s.nextLine();
if(courseCode.equals("END"))
{
continue;
}
if(courseCode.length() <= 8)
{
for(int i=0; i<4;i++)
{
if(Character.isLetter(courseCode.charAt(i)))
{
temp+=courseCode.charAt(i);
}
else
{
throw new CourseCodeException("Unacceptable Computing Course Code");
}
}
if(Character.isDigit(courseCode.charAt(4)))
{
if(Character.getNumericValue(courseCode.charAt(4))>=1 && Character.getNumericValue(courseCode.charAt(4))<=3)
{
temp+=courseCode.charAt(4);
}
else
{
throw new CourseCodeException("Unacceptable Computing Course Code");
}
}
else
{
throw new CourseCodeException("Unacceptable Computing Course Code");
}
for(int i=5; i<=7;i++)
{
if(Character.isDigit(courseCode.charAt(i)))
{
temp+=courseCode.charAt(i);
}
else
{
throw new CourseCodeException("Unacceptable Computing Course Code");
}
}
BufferedWriter writer = new BufferedWriter(new FileWriter("C:\\ValidCode.txt"));
writer.write(temp);
writer.newLine();
writer.close();
}
else
{
throw new CourseCodeException("Unacceptable Computing Course Code");
}
}
catch(Exception ex)
{
System.out.print(ex+"\n\n");
errorCount++;
continue;
}
try
{
System.out.println("Enter number of credits: ");
credit = Integer.parseInt(s.nextLine());
}
catch(NumberFormatException ex)
{
System.out.print("Invalid Credit Input!\n\n");
}
}
}
}