This is a casino number guessing game written using the C++ programming language. The program start off by asking the user for his/her name and the deposited amount needed to play the game. The program then prompt the user to guess a number from 1 to 10, if the user guesses correctly they get ten (10) times the amount bet. If the user guess incorrectly they lose the betting amount.
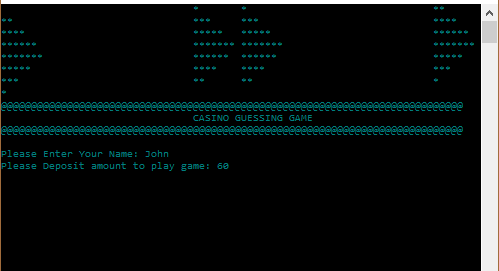
Code:
#include <iostream>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
void main_menu();
int main()
{
string name;
int amt;
int betAmt;
int g;
int comp;
char options;
srand(time(0));
system("cls");
system("color 03");
cout<<"\t\t\t\t*\t*";
cout<<"\t\t\t\t**\t**";
cout<<"\t\t\t\t***\t***";
cout<<"\t\t\t\t****\t****";
cout<<"\t\t\t\t*****\t*****";
cout<<"\t\t\t\t******\t******";
cout<<"\t\t\t\t*******\t*******";
cout<<"\t\t\t\t*******\t*******";
cout<<"\t\t\t\t******\t******";
cout<<"\t\t\t\t*****\t*****";
cout<<"\t\t\t\t****\t****";
cout<<"\t\t\t\t***\t***";
cout<<"\t\t\t\t**\t**";
cout<<"\t\t\t\t*\t*";
cout << "\n@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@";
cout << "\n\t\t\t\tCASINO GUESSING GAME\n";
cout << "@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@\n\n";
cout << "Please Enter Your Name: ";
getline(cin, name);
cout << "Please Deposit amount to play game: ";
cin >> amt;
do
{
system("cls");
main_menu();
cout << "\n\nYour current balance is $ " << amt << "\n";
do
{
cout <<name<<" enter money to bet : $";
cin >> betAmt;
if(betAmt > amt)
cout << "Your betting amount is more than your current balance\n"
<<"\nRe-enter data\n ";
}while(betAmt > amt);
do
{
cout << "Choose a number from 1 to 10 :";
cin >> g;
if(g <= 0 || g > 10)
cout << "Please check the number!! should be between 1 to 10\n"
<<"\nRe-enter data\n ";
}while(g <= 0 || g > 10);
comp = rand()%10 + 1;
if(comp == g)
{
cout << "\n\nGood Luck!! You won $" << betAmt * 10;
amt = amt + betAmt * 10;
}
else
{
cout << "Better Luck Next Time!! You lost $ "<< betAmt <<"\n";
amt = amt - betAmt;
}
cout << "\nThe winning number was : " << comp <<"\n";
cout << "\n"<<name<<", You have $ " << amt << "\n";
if(amt == 0)
{
cout << "You have no money to play ";
break;
}
cout << "\n\nDo you want to play again (y/n)? ";
cin >> options;
}while(options =='Y'|| options=='y');
cout << "\n";
cout << "@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@";
cout << "\nThanks for playing game. Your balance amt is $ " << amt << "\n";
cout << "@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@";
return 0;
}
void main_menu()
{
system("cls");
cout << "\n";
cout << "\t\tInstuctions\n";
cout << "@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@\n";
cout << "\t1. Choose any number between 1 to 10\n";
cout << "\t2. If you win you will get 10 times the money you bet\n";
cout << "\t3. If you bet the wrong number you will lose your betting amount\n\n";
cout << "@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@\n";
}