This is a Rock Paper Scissors Game that was programmed using the visual basic programming language. In this game the player plays against the computer, the player choose rock, paper or scissors from the option, then the computer rondomly choose from the same options. Based on what is selected by both players, the player determines who is the victor.
If the same item is selected, it's a tie. If a rock and scissors are selected, the rock wins, because a rock can smash scissors. If scissors and paper are selected, the scissors win, because scissors can cut paper. If paper and a rock are selected, the paper wins, because a sheet of paper can cover a rock.
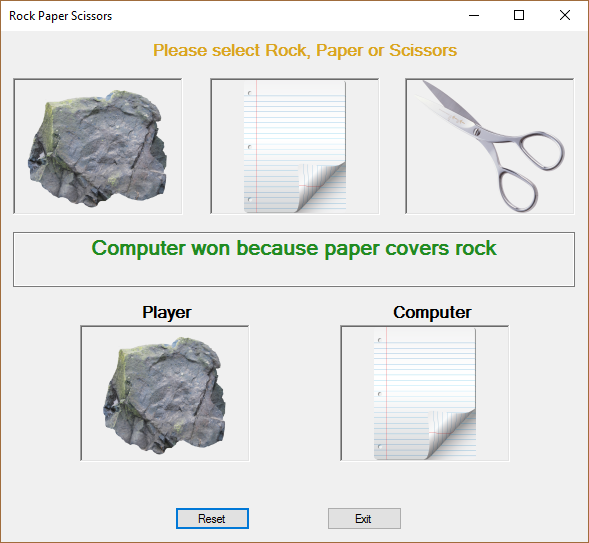
Public Class frmMain
Dim PictureBox1 As New PictureBox
Private Sub btnRock_Click(sender As Object, e As EventArgs) Handles btnRock.Click
Dim randomGenerator As New Random()
Dim computerChoice As Integer
picPlayer.Image = btnRock.Image
computerChoice = randomGenerator.[Next](1, 4)
Select Case computerChoice
Case 1
picComputer.Image = btnRock.Image
txtWinner.Text = " TIE "
Exit Select
Case 2
picComputer.Image = btnPaper.Image
txtWinner.Text = " Computer won because paper covers rock "
Exit Select
Case 3
picComputer.Image = btnScissors.Image
txtWinner.Text = " Player won because rock breaks scissors "
Exit Select
End Select
End Sub
Private Sub btnPaper_Click(sender As Object, e As EventArgs) Handles btnPaper.Click
Dim randomGenerator As New Random()
Dim computerChoice As Integer
picPlayer.Image = btnPaper.Image
computerChoice = randomGenerator.[Next](1, 4)
Select Case computerChoice
Case 1
picComputer.Image = btnRock.Image
txtWinner.Text = " Player won because paper covers rock "
Exit Select
Case 2
picComputer.Image = btnPaper.Image
txtWinner.Text = " TIE "
Exit Select
Case 3
picComputer.Image = btnScissors.Image
txtWinner.Text = " Computer won because scissors cut paper "
Exit Select
End Select
End Sub
Private Sub btnScissors_Click(sender As Object, e As EventArgs) Handles btnScissors.Click
Dim randomGenerator As New Random()
Dim computerChoice As Integer
picPlayer.Image = btnScissors.Image
computerChoice = randomGenerator.[Next](1, 4)
Select Case computerChoice
Case 1
picComputer.Image = btnRock.Image
txtWinner.Text = " Computer won because rock breaks scissors "
Exit Select
Case 2
picComputer.Image = btnPaper.Image
txtWinner.Text = " Player won because scissors cut paper "
Exit Select
Case 3
picComputer.Image = btnScissors.Image
txtWinner.Text = " TIE "
Exit Select
End Select
End Sub
Private Sub btnReset_Click(sender As Object, e As EventArgs) Handles btnReset.Click
picComputer.Image = PictureBox1.Image
picPlayer.Image = PictureBox1.Image
End Sub
Private Sub frmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load
PictureBox1.BackColor = SystemColors.Control
End Sub
Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
Me.Close()
End Sub
End Class