Hangman is a classic word-guessing game for one or more players. It is used often by teachers to practice spelling, vocabulary and just for fun. In this hangman game the user is given a few letters out of the word, in order to complete the game the user have to guess all the other letters in the word. If the user guess all the letters correctly they will win the game, on the other hand if the amount of try runs out they lose the game. The entire word will be revealed if they lose the game. The user has an option to create a new game when the game starts and an option to quit or reset while the game is in play.
In order for the game to run, a list of words must be added to the application executable folder.
Example:
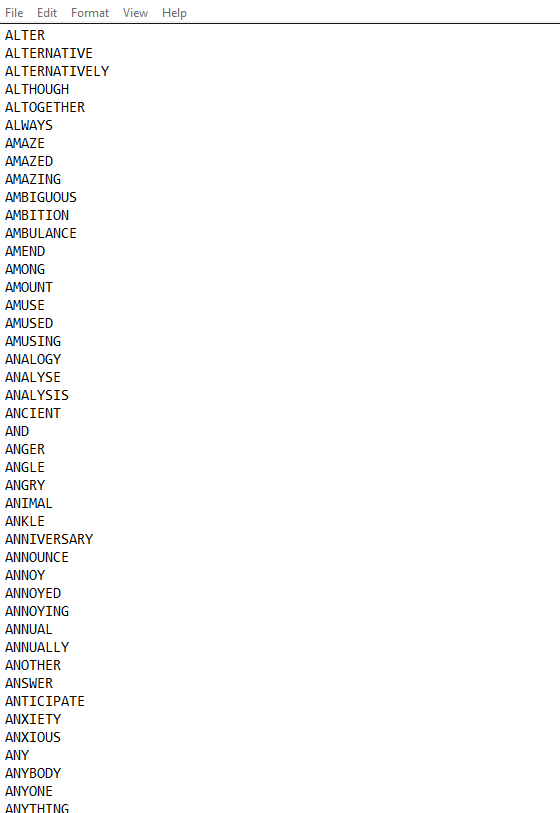
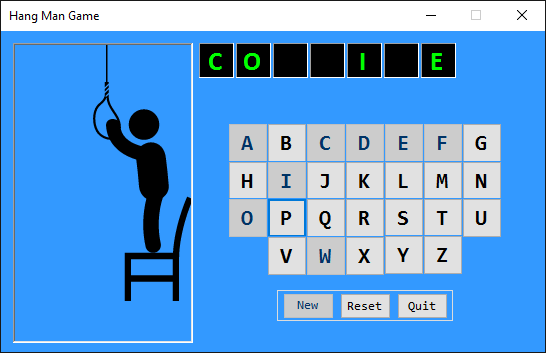
WordHolder Class:
class WordHolder
{
public int Start { get; set; }
public int End { get; set; }
private List<WordHolder> _slotCollection = null;
public List<WordHolder> SlotCollection { get { return _slotCollection; } }
public WordHolder() { }
public WordHolder(int count)
{
WordHolder obj = null;
_slotCollection = new List<WordHolder>();
if (count < 1000)
{
SlotCollection.Add(new WordHolder() { Start = 0, End = count });
return;
}
else
{
int endValue = 0;
for (int i = 0; i < count; i += 1000)
{
obj = new WordHolder()
{
Start = i == 0 ? i : i + 1,
End = count - endValue > 1000 ? (1000 + endValue) : i + (count - endValue)
};
_slotCollection.Add(obj);
endValue += 1000;
}
}
}
}
Main Code:
using System;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Windows.Forms;
namespace HangMan
{
public partial class frmHangmanGame : Form
{
private StreamReader _readit = null;
private WordHolder _wordHolder = null;
private String[] _wordListArray = null;
private Char[] _wordArray = null;
private Char[] _display = null;
private int[] _randomCollection = null;
private Random _random = null;
private String _randomWord = String.Empty;
private int _wordIndex = 0;
private int _previousNumber = 0;
private int _imageIndex = 0;
private int _remain = 0;
private int _slotCount = 0;
private int _outerCount = 0;
private int _innerCount = 0;
private Boolean _isQuit = false;
private KeysConverter _converter = null;
public frmHangmanGame()
{
InitializeComponent();
}
private void FormHang_Load(object sender, EventArgs e)
{
_random = new Random();
_converter = new KeysConverter();
this.LoadWordBox();
this.Reset();
}
private void FormHang_Shown(object sender, EventArgs e)
{
this.Cursor = Cursors.WaitCursor;
try
{
this.LoadWordList();
_wordHolder = new WordHolder(_wordListArray.Length);
_slotCount = _wordHolder.SlotCollection.Count;
}
catch (Exception ex)
{
buttonReset.Enabled = false;
buttonNewGame.Enabled = false;
MessageBox.Show(ex.Message.StartsWith("Could not find file") == true ?
"Please Copy the list of words file into application executable folder." : ex.Message,
this.Text, MessageBoxButtons.OK, MessageBoxIcon.Error);
}
this.Cursor = Cursors.Default;
}
private void buttonAbandon_Click(object sender, EventArgs e)
{
this.GameQuit();
}
private void buttonReset_Click(object sender, EventArgs e)
{
this.Reset();
buttonQuit.Enabled = false;
buttonNewGame.Enabled = true;
}
private void buttonNewGame_Click(object sender, EventArgs e)
{
try
{
if (_wordListArray.Length == 0)
{
MessageBox.Show("Dictionary not found.",
this.Text,
MessageBoxButtons.OK, MessageBoxIcon.Error);
Application.ExitThread();
Application.Exit();
return;
}
this.Reset();
int showValues = 0;
if (_outerCount >= _slotCount)
{
_outerCount = 0;
_innerCount = 0;
_randomCollection = null;
_randomCollection = new int[1];
}
WordHolder obj = new WordHolder();
obj.Start = _wordHolder.SlotCollection[_outerCount].Start;
obj.End = _wordHolder.SlotCollection[_outerCount].End;
bool result = true;
if (_randomCollection == null) _randomCollection = new int[1];
while (result)
{
result = true;
_wordIndex = _random.Next(obj.Start, obj.End - 1);
var isfound = (from n in _randomCollection
where n == _wordIndex
select n).Count();
if (isfound <= 0)
{
if (_randomCollection.Length == 1)
_randomCollection[_randomCollection.Length - 1] = _wordIndex;
_randomCollection[_randomCollection.Length - 1] = _wordIndex;
Array.Resize(ref _randomCollection, _randomCollection.Length + 1);
result = false;
}
}
_innerCount += 1;
if (_innerCount >= 10)
{
_innerCount = 0;
_outerCount += 1;
_randomCollection = null;
_randomCollection = new int[1];
}
_previousNumber = _wordIndex;
_randomWord = _wordListArray[_wordIndex].ToUpper();
_wordArray = _randomWord.ToCharArray();
this.CreateLabelBox(_wordArray.Length);
foreach (Control control in this.Controls)
if (control is Button) control.Enabled = true;
switch (_wordArray.Length)
{
case 1:
case 2:
case 3:
case 4:
showValues = 1;
break;
case 5:
case 6:
case 7:
case 8:
showValues = 2;
break;
default:
showValues = 3;
break;
}
Control.ControlCollection collection = this.Controls;
_display = new Char[showValues];
foreach (Control control in collection)
{
if (control is Label)
switch (showValues)
{
case 1:
if (control.Name == String.Format("{0}{1}", "labelWord", "01"))
{
control.Text = _wordArray[0].ToString();
_display[0] = Char.Parse(control.Text);
}
break;
case 2:
if (control.Name == String.Format("{0}{1}", "labelWord", "01"))
{
control.Text = _wordArray[0].ToString();
_display[0] = Char.Parse(control.Text);
}
if (control.Name == String.Format("{0}{1}", "labelWord", (_wordArray.Length).ToString("00")))
{
control.Text = _wordArray[_wordArray.Length - 1].ToString();
_display[1] = Char.Parse(control.Text);
}
break;
case 3:
if (control.Name == String.Format("{0}{1}", "labelWord", "01"))
{
control.Text = _wordArray[0].ToString();
_display[0] = Char.Parse(control.Text);
}
int middle = _wordArray.Length / 2;
if (control.Name == String.Format("{0}{1}", "labelWord", middle.ToString("00")))
{
control.Text = _wordArray[middle - 1].ToString();
_display[1] = Char.Parse(control.Text);
}
if (control.Name == String.Format("{0}{1}", "labelWord", (_wordArray.Length).ToString("00")))
{
control.Text = _wordArray[_wordArray.Length - 1].ToString();
_display[2] = Char.Parse(control.Text);
}
break;
}
}
Array.Sort(_display);
char value = '@';
foreach (Char charvalue in _display)
{
if (value == charvalue)continue;
value = charvalue;
int innerCount = (from c in _display
where c == charvalue
select c).Count();
int outerCount = (from c in _wordArray
where c == charvalue
select c).Count();
if (outerCount - innerCount <= 0)
{
foreach (Control control in collection)
{
if (control is Button)
{
if (control.Name == String.Format("{0}{1}", "button", value))
control.Enabled = false;
}
}
}
}
buttonQuit.Enabled = true;
buttonNewGame.Enabled = false;
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, this.Text, MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
private void GameQuit()
{
_isQuit = true;
this.GameStatus(true);
}
private void Reset()
{
pictureBoxHangMan.Image = null;
foreach (Control control in this.Controls)
{
if (control is Label)
{
control.ForeColor = Color.Lime;
control.Visible = false;
control.ResetText();
}
if (control is Button) control.Enabled = false;
}
_outerCount = 0;
_innerCount = 0;
_randomCollection = null;
_wordIndex = 0;
_imageIndex = 0;
_isQuit = false;
_wordArray = null;
buttonQuit.Enabled = false;
}
private void ShowHangMan(int position)
{
switch (position)
{
case 1:
pictureBoxHangMan.Image = Properties.Resources.IMG_01;
break;
case 2:
pictureBoxHangMan.Image = Properties.Resources.IMG_02;
break;
case 3:
pictureBoxHangMan.Image = Properties.Resources.IMG_03;
break;
case 4:
pictureBoxHangMan.Image = Properties.Resources.IMG_04;
break;
case 5:
pictureBoxHangMan.Image = Properties.Resources.IMG_05;
break;
case 6:
pictureBoxHangMan.Image = Properties.Resources.IMG_06;
break;
case 7:
pictureBoxHangMan.Image = Properties.Resources.IMG_07;
break;
case 8:
pictureBoxHangMan.Image = Properties.Resources.IMG_08;
break;
case 9:
pictureBoxHangMan.Image = Properties.Resources.IMG_FINAL;
break;
default:
pictureBoxHangMan.Image = null;
break;
}
}
private void TextButton(object sender, EventArgs e)
{
Button btn = sender as Button;
String btnText = btn.Text;
this.SetGame(btnText);
}
private void LoadWordList()
{
_readit = new StreamReader(File.Open("listOfWords.txt", FileMode.Open, FileAccess.Read));
int count = 0;
_wordListArray = new String[1];
string line = string.Empty;
while (_readit.Peek() != -1)
{
Application.DoEvents();
line = _readit.ReadLine().Trim();
if (line.Length >= 19)
continue;
count += 1;
Array.Resize(ref _wordListArray, count);
_wordListArray[count - 1] = line;
}
_readit.Close();
_readit.Dispose();
_readit = null;
}
private void LoadWordBox()
{
int lineOne = 198;
int lineTwo = 198;
for (int i = 1; i <= 18; i++)
{
String labelName = String.Format("{0}{1}", "labelWord", i.ToString("00"));
Control ctrl = new Control();
Label word = new Label();
word.Name = String.Format("{0}{1}", "labelWord", i.ToString("00"));
word.Size = new Size(35, 35);
word.TextAlign = ContentAlignment.MiddleCenter;
word.BackColor = Color.Black;
word.ForeColor = Color.Lime;
word.Font = new Font("Calibri", 20, FontStyle.Bold);
if (i <= 9)
{
word.Location = new Point(lineOne, 12);
lineOne += 37;
}
if (i >= 10)
{
word.Location = new Point(lineTwo, 47);
lineTwo += 37;
}
word.Text = String.Empty;
word.BorderStyle = BorderStyle.Fixed3D;
word.Visible = true;
this.Controls.Add(word);
}
}
private void CreateLabelBox(int count)
{
int index = 1;
Control.ControlCollection collection = this.Controls;
foreach (Control control in collection)
if (control is Label)
if (control.Name == String.Format("{0}{1}", "labelWord", index.ToString("00")))
{
control.Font = new Font("Calibri", 20, FontStyle.Bold);
control.ResetText();
control.Visible = true;
index += 1;
if (index > count) return;
}
}
private void GameStatus(Boolean islost)
{
if (islost) pictureBoxHangMan.Image = Properties.Resources.IMG_FINAL;
_wordIndex = 0;
foreach (Control control in this.Controls)
{
if (control is Label)
if (control.Name != "myLabel")
{
if ((String.IsNullOrEmpty(control.Text)) && (control.Visible == true))
{
control.Font = new Font("Calibri", 20, FontStyle.Bold);
control.ForeColor = Color.DarkSeaGreen;
control.Text = _wordArray[_wordIndex].ToString();
}
if ((!String.IsNullOrEmpty(control.Text)) && (control.Visible == true)) _wordIndex += 1;
}
if (control is Button) control.Enabled = false;
}
buttonQuit.Enabled = false;
buttonNewGame.Enabled = true;
}
private void SetGame(String word)
{
int result = (from w in _wordArray
where w == Char.Parse(word)
select w).Count();
int count = 0;
int[] indexArray = new int[1];
for (int i = 0; i < _wordArray.Length; i++)
{
if (_wordArray[i] == Char.Parse(word))
{
count += 1;
Array.Resize(ref indexArray, count);
indexArray[count - 1] = i;
}
}
_remain = 0;
Control.ControlCollection collection = this.Controls;
foreach (Control control in collection)
{
if (control is Label)
if (control.Name != "myLabel")
{
foreach (int index in indexArray)
{
if (control.Name == String.Format("{0}{1}", "labelWord", (index + 1).ToString("00")) && control.Visible)
{
if (String.IsNullOrEmpty(control.Text))
control.Text = word;
}
}
if (String.IsNullOrEmpty(control.Text) && control.Visible)
_remain += 1;
}
if (control is Button)
{
if (control.Name == String.Format("{0}{1}", "button", word.ToUpper()))
{
if (!control.Enabled) return;
control.Enabled = false;
}
}
}
if (result == 0)
{
_imageIndex += 1;
ShowHangMan(_imageIndex);
if (_imageIndex >= 9) GameStatus(true);
return;
}
if (_remain <= 0)
{
MessageBox.Show("Congratulations! You Successfully Won.",
this.Text, MessageBoxButtons.OK,
MessageBoxIcon.Information);
GameStatus(false);
}
}
}
}