The Ministry of Labor has asked you to create a program that uses a VECTOR of counters that will help them to determine how many salespersons from Mitch’s Shipping Service earn salaries in each of the following ranges:
Ranges
|
$600-$699
|
$700-$799
|
$800-$899
|
$900-$999
|
$1000 and over
|
This company pays its salespersons on a commission basis. You are required to create a function called calCommission, which returns an integer value, and accepts an integer argument for the gross sales. This function should calculate the salespersons’ weekly salary; you were told that each receive $200 per week plus 10 percent of their gross sales for that week. For example, a salesperson who grosses $5000 in sales in a week receives $200 plus 10 percent of $5000, or a total of $700.
Additional Functions:
printSummary(): This function displays a summary of the number of salespersons being paid at a particular range.
updateArray(int): This function accepts as argument the salesperson’s Commission and updates the vector of counters accordingly.
SAMPLE DATA
Salesperson
|
Gross Sales
|
Commission
|
1
|
$5000
|
$700
|
2
|
$10000
|
$1200
|
3
|
$6000
|
$800
|
4
|
$4000
|
$600
|
5
|
$20000
|
$2200
|
Ranges
|
Array of Counters
|
$600-$699
|
1
|
$700-$799
|
1
|
$800-$899
|
1
|
$900-$999
|
0
|
$1000 and over
|
2
|
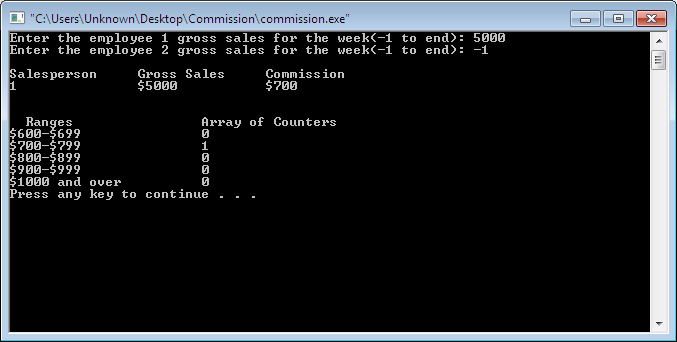
#include <iostream>
#include <vector>
using namespace std;
int calCommission(int gross_sales);
void printSummary();
void updateArray(int Commission);
vector<int> hold_gross_sales;
vector<int> commission;
int main(int argc, char *argv[])
{
int gross_sales;
int salery;
int counter = 0;
while(gross_sales != -1)
{
cout<<"Enter the employee "<<counter+1<<" gross sales for the week(-1 to end): ";
cin>> gross_sales;
if(gross_sales != -1)
{
salery = calCommission(gross_sales);
updateArray(salery);
hold_gross_sales.push_back(gross_sales);
counter++;
}
}
cout<<endl<<"Salesperson\tGross Sales\tCommission"<<endl;
for(int i = 0; i < commission.size(); i++)
{
cout<<i+1<<"\t\t"<<"$"<<hold_gross_sales[i]<<"\t\t"<<"$"<<commission[i]<<endl<<endl;
}
printSummary();
return 0;
}
int calCommission(int gross_sales)
{
return (0.1 * gross_sales) + 200;
}
void printSummary()
{
int value600to699 = 0;
int value700to799 = 0;
int value800to899 = 0;
int value900to999 = 0;
int value1000Greater = 0;
for(int i = 0; i < commission.size(); i++)
{
if(commission[i] >= 600 && commission[i] <= 699)
{
value600to699++;
}
else if(commission[i] >= 700 && commission[i] <= 799)
{
value700to799++;
}
else if(commission[i] >= 800 && commission[i] <= 899)
{
value800to899++;
}
else if(commission[i] >= 900 && commission[i] <= 999)
{
value900to999++;
}
else if(commission[i] >= 1000)
{
value1000Greater++;
}
}
cout<<endl<<" Ranges\t\tArray of Counters"<<endl;
cout<<"$600-$699"<<"\t\t"<<value600to699<<endl;
cout<<"$700-$799"<<"\t\t"<<value700to799<<endl;
cout<<"$800-$899"<<"\t\t"<<value800to899<<endl;
cout<<"$900-$999"<<"\t\t"<<value900to999<<endl;
cout<<"$1000 and over"<<"\t\t"<<value1000Greater<<endl;
}
void updateArray(int Commission)
{
commission.push_back(Commission);
}