A transpose of an array is obtained by interchanging the elements of rows and columns. A class Transarray contains a two dimensional integer array of order [ m x n]. The maximum value possible for both ‘m’ and ‘n’ is 20. Design a class Transarray to find the transpose of a given matrix. The details of the members of the class are given below:
Class name : Transarray
Data members
arr[][] : Stores the matrix elements
m : Integer to store the number of rows.
n : Integer to store the number of columns.
Member functions
Transarray() : Default constructor.
Transarray(intmm,intnn) : to initialize the size of the matrix, m=mm, n=nn.
Void fillarray() : to enter elements into the matrix
void transpose(Transarray A) : to find the transpose of a given matrix.
voiddisaparray() : displays the array in a matrix form.
Output:
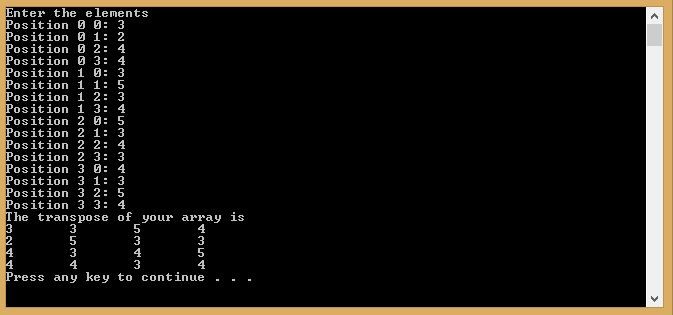
Code:
#include <iostream>
#include <string>
#include <cstdlib>
using namespace std;
class Transarray
{
private:
int arr[20][20];
int m,n;
public:
Transarray();
Transarray(int mm, int nn);
void fillarray();
void transpose(Transarray A);
void disparray();
};
Transarray::Transarray()
{
m = 5;
n = 5;
}
Transarray::Transarray(int mm, int nn)
{
m = mm;
n = nn;
}
void Transarray::fillarray()
{
cout<<"Enter the elements "<<endl;
for(int i = 0; i < m; i++)
{
for(int j = 0; j < n; j++)
{
cout<<"Position "<<i <<" "<<j <<": ";
cin>>arr[i][j];
}
}
}
void Transarray::transpose(Transarray A)
{
m = A.n;
n = A.m;
for (int i = 0; i<m; i++)
{
for (int j = 0; j<n; j++)
{
arr[i][j] = A.arr[j][i];
}
}
}
void Transarray::disparray()
{
cout<<"The transpose of your array is "<<endl;
for(int i = 0; i < m; i++)
{
for(int j = 0;j < n; j++)
{
cout<<arr[i][j]<<"\t";
}
cout<<endl;
}
}
int main()
{
Transarray T1(4,4);
Transarray T2;
T1.fillarray();
T2.transpose (T1);
T2.disparray();
system("pause");
return 0;
}