This program removes the virus that creates shortcuts in USB Drive and computer.
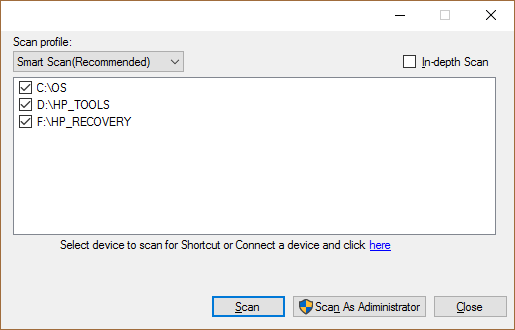
Code:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Runtime.InteropServices;
using System.Drawing;
using System.Xml.Linq;
using System.Security.Principal ;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using Microsoft.Win32;
using System.Diagnostics;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
[DllImport("user32.dll")]
public static extern IntPtr SendMessage(IntPtr hwnd, uint msg, uint wParam, uint lParam);
const Int32 BTN_SHIELD = 0x160C;
Int32 ErrorCount=0;
List<string> ListOfVirusToScanFor,ErrorList;
List<string> VirusPaths = new List<string>();
List<string> VbList;
string savecheckedfiles=Path.GetDirectoryName(Application.ExecutablePath) + "\\chki.txt";
Boolean wsRunning, ORunning=false ;
public static void AddShieldToScanAsButton(Button btn)
{
SendMessage(btn.Handle, BTN_SHIELD, 0, 0xFFFFFFFF);
}
private void Form1_Load(object sender, EventArgs e)
{
ErrorList = new List<string>();
//get all connected drives in this computer
foreach (string USBorHdd in Environment.GetLogicalDrives())
{
//check if drive can be used
if (new DriveInfo(USBorHdd).IsReady == true)
{
//in this version, we wont scan cd/dvd
if (new DriveInfo(USBorHdd).DriveType != DriveType.CDRom)
{
try
{
ListView1.Items.Add(USBorHdd + new DriveInfo(USBorHdd).VolumeLabel);
}
catch (Exception ex)
{
ErrorList.Add(ex.Message);
}
}
}
}
//check if current user account is the member of administrator
WindowsIdentity Windentity = WindowsIdentity.GetCurrent ();
WindowsPrincipal wPrincipal = new WindowsPrincipal(Windentity);
if (wPrincipal.IsInRole(WindowsBuiltInRole.Administrator) == true)
{
ButtonScanAs.Visible = false;
//relocate scan and close button
buttonScan.Location = new Point(buttonScan.Location.X + 95, buttonScan.Location.Y);
buttonClose.Location = new Point(buttonClose.Location.X - 20, buttonClose.Location.Y);
if (File.Exists(savecheckedfiles) == true)
{
foreach (string line in File.ReadAllLines(savecheckedfiles))
{
for (Int32 i = 0; i < ListView1.Items.Count - 1; i++)
{
if (ListView1.Items[i].Text == line)
{
ListView1.Items[i].Checked = true;
}
}
}
}
else
{
ComboBox1.SelectedIndex = 0;
}
}
else
{
//Add shield in scan as button
AddShieldToScanAsButton(ButtonScanAs);
//select the first item in the combobox
ComboBox1.SelectedIndex = 0;
}
}
private void buttonScan_Click(object sender, EventArgs e)
{
ListOfVirusToScanFor = new List<string>(new string[] { "new folder.exe", "newfolder.exe","srvidd20.exe","iddono20.exe","editor.exe", "doieuln.dll", "svchost .exe", "scvshosts.exe", "scvhsot.exe", "scvvhsot.exe", "hinhem.scr", "regsvr.exe", "blastclnnn.exe", "autorun.ini" });
VbList = new List<string>(new string[] { "*.vbs", "*.vbe" });
ListView1.Enabled = buttonScan.Enabled = buttonView.Visible = false;
LabelNotification.Text = "Getting processes and check processes.";
foreach (Process Wscript in Process.GetProcesses())
{
//check if wscript is running
if (Wscript.ProcessName.ToLower () == "wscript")
{
wsRunning = true;
}
//this is virus name its like this tmp####.tmp.exe
if ((Wscript.ProcessName.StartsWith("tmp") == true) && (Wscript.ProcessName.EndsWith(".tmp") == true))
{
VirusPaths.Add(Wscript.MainModule.FileName);
ORunning = true;
}
foreach (string VirusInList in ListOfVirusToScanFor.ToArray())
{
if (Wscript.ProcessName.ToLower() == VirusInList)
{
ORunning = true;
}
}
}
if (wsRunning == true)
{
foreach (Process currentRunningProcess in Process.GetProcessesByName("wscript"))
{
LabelNotification.Text = "Ending process.";
Retry:
bool FailedError = false;
try
{
//close wscript, so that we can unhide files that hidden by the wscript
currentRunningProcess.Kill();
LabelNotification.Text = "Process was ended successfully";
}
catch (Exception ex)
{
ErrorCount++;
if (ErrorCount == 2)
{
MessageBox.Show(ex.Message + "This application is going to close, and try restarting your computer and run this application again, Sorry");
//start windows explorer
Process.Start("Explorer.exe");
this.Close();
}
FailedError = true;
}
if (FailedError == true)
{
//get explorer in current running process
foreach (Process XplorerRunningProcess in Process.GetProcessesByName("explorer"))
{
try
{
//close windows explorer
XplorerRunningProcess.Kill();
goto Retry;
}catch (Exception ex)
{
ErrorList.Add("Failed to end process '" + Path.GetFileName(XplorerRunningProcess.MainModule.FileName) + "' because " + ex.Message);
}
}
}
}
LabelNotification.Text = "Scanning Drives";
/*check in startup folder and in temporary folder
'Winlog virus */
foreach (string VbsFile in Directory .EnumerateFiles (Environment.GetFolderPath(Environment.SpecialFolder.Startup),"*.vbs"))
{
if (File.Exists(VbsFile) == true)
{
VirusPaths.Add( VbsFile );
}
}
foreach (string vbscript in VbList.ToArray())
{
foreach (string vbsfile in (Directory.EnumerateFiles(Path.GetTempPath(), vbscript)))
{
if (File.Exists(vbsfile) == true)
{
VirusPaths.Add(vbsfile);
}
}
//search for vbs files in ther user profile folder
foreach (string vfile in Directory.EnumerateFiles(System.Environment.GetEnvironmentVariable("USERPROFILE"), vbscript))
{
VirusPaths.Add(vfile);
}
//get the drives checked
foreach (ListViewItem Checkeditems in ListView1.CheckedItems)
{
string USBorHdd = Checkeditems.Text;
//get path root
string DriveName = USBorHdd.Substring(0, 3);
bool isBusy = new DriveInfo(DriveName).IsReady;
//check if drive can be used
if (isBusy == true)
{
foreach (string vbsfile in Directory.EnumerateFiles(DriveName, vbscript))
{
if (File.Exists(vbsfile) == true)
{
VirusPaths.Add(vbsfile);
}
}
}
}
}
}
else if (ORunning == true)
{
LabelNotification.Text = "Please wait...";
foreach (string VinList in ListOfVirusToScanFor.ToArray())
{
string Vlist;
Vlist = VinList.Remove(VinList.Length - 4, 4);
foreach (Process curProc in Process.GetProcessesByName(Vlist))
{
//check if path exist
if (File.Exists(curProc.MainModule.FileName) == true)
{
//add file path into the list
VirusPaths.Add(curProc.MainModule.FileName);
}
try
{
//end process
curProc.Kill();
}
catch (Exception ex)
{
ErrorList.Add("Failed to end process '" + Path.GetFileName(curProc.MainModule.FileName) + "' because " + ex.Message);
}
}
}
//check if current user account is the member of administrator
WindowsIdentity Windentity = WindowsIdentity.GetCurrent ();
WindowsPrincipal wPrincipal = new WindowsPrincipal(Windentity);
if (wPrincipal.IsInRole(WindowsBuiltInRole.Administrator) == true)
{
List <string > SuspectedPath=new List<string> (new string[] {Environment.SystemDirectory, Environment.GetEnvironmentVariable("WINDIR"), Environment.GetEnvironmentVariable("APPDATA"), Environment.GetEnvironmentVariable("TEMP"), Environment.GetFolderPath(Environment.SpecialFolder.Startup), System.Environment.GetEnvironmentVariable("USERPROFILE")});
RegistryKey regkey=Registry.LocalMachine.OpenSubKey("Software\\Microsoft\\Windows\\CurrentVersion\\Run", true);
foreach (string Startupfiles in regkey.GetValueNames())
{
foreach (string vinlist in ListOfVirusToScanFor.ToArray())
{
if (Startupfiles.Contains(vinlist) == true)
{
if (File.Exists(regkey.GetValue(Startupfiles).ToString()) == true)
{
VirusPaths.Add(regkey.GetValue(Startupfiles).ToString());
try
{
regkey.DeleteValue(regkey.GetValue(Startupfiles).ToString());
}
catch (Exception ex)
{
ErrorList.Add("Failed deleting '" + regkey.GetValue(Startupfiles ).ToString() + "' because " + ex.Message);
}
}
}
else if (Startupfiles.StartsWith("rundll32.exe") == true)
{
VirusPaths.Add(regkey.GetValue(Startupfiles).ToString());
try
{
regkey.DeleteValue(regkey.GetValue(Startupfiles).ToString());
}
catch (Exception ex)
{
ErrorList.Add("Failed deleting '" + regkey.GetValue(Startupfiles).ToString() + "' because " + ex.Message);
}
}
else if (Startupfiles.Contains("ServiceDll") == true)
{
VirusPaths.Add(regkey.GetValue(Startupfiles).ToString());
try
{
regkey.DeleteValue(regkey.GetValue(Startupfiles).ToString());
}
catch (Exception ex)
{
ErrorList.Add("Failed deleting '" + regkey.GetValue(Startupfiles).ToString() + "' because " + ex.Message);
}
}
}
//This virus disable task manager, regedit, no folder option
//Enable Task manager, regedit, no folder option in the LocalMachine
regkey =Registry.CurrentUser.OpenSubKey("Software\\Microsoft\\Windows\\CurrentVersion\\Policies\\System", true);
regkey.SetValue("DisableTaskMgr", 0, RegistryValueKind.DWord);
regkey.SetValue("DisableRegistryTools", 0, RegistryValueKind.DWord);
regkey.SetValue("NoFolderOptions", 0, RegistryValueKind.DWord);
regkey.SetValue("Hidden", 1, RegistryValueKind.DWord);
regkey.Close();
//open registry
regkey = Registry.CurrentUser.OpenSubKey("Software\\Microsoft\\Windows\\CurrentVersion\\Run", true);
foreach (string startupfiles in regkey.GetValueNames())
{
foreach (string vinlist in ListOfVirusToScanFor.ToArray())
{
if (startupfiles.Contains(vinlist) == true)
{
if (File.Exists(regkey.GetValue(startupfiles).ToString()) == true)
{
VirusPaths.Add(regkey.GetValue(startupfiles).ToString());
try
{
regkey.DeleteValue(regkey.GetValue(startupfiles).ToString());
}
catch (Exception ex)
{
ErrorList.Add("Failed deleting '" + regkey.GetValue(startupfiles).ToString() + "' because " + ex.Message);
}
}
}
else if (startupfiles.StartsWith("rundll32.exe") == true)
{
VirusPaths.Add(regkey.GetValue(startupfiles).ToString());
try
{
regkey.DeleteValue(regkey.GetValue(startupfiles).ToString());
}
catch (Exception ex)
{
ErrorList.Add("Failed deleting '" + regkey.GetValue(startupfiles).ToString() + "' because " + ex.Message);
}
}
else if (startupfiles.Contains("ServiceDll") == true)
{
VirusPaths.Add(regkey.GetValue(startupfiles).ToString());
try
{
regkey.DeleteValue(regkey.GetValue(startupfiles).ToString());
}
catch (Exception ex)
{
ErrorList.Add("Failed deleting '" + regkey.GetValue(startupfiles).ToString() + "' because " + ex.Message);
}
}
}
}
//for vista and later operating system, the malware changes the global setting for TCP Receive Window Autotuning to disabled
//so to change it back
Process.Start("cmd.exe netsh interface tcp set global autotuninglevel=normal");
regkey =Registry.CurrentUser.OpenSubKey("Software\\Microsoft\\Windows\\CurrentVersion\\Policies\\System", true);
regkey.SetValue("DisableTaskMgr", 0, RegistryValueKind.DWord);
regkey.SetValue("DisableRegistryTools", 0, RegistryValueKind.DWord);
regkey.SetValue("NoFolderOptions", 0, RegistryValueKind.DWord);
regkey.SetValue("Hidden", 1, RegistryValueKind.DWord);
regkey = Registry.LocalMachine.OpenSubKey("Software\\Microsoft\\Windows NT\\CurrentVersion\\Winlogon", true);
regkey.SetValue("Shell", "explorer.exe");
LabelNotification.Text = "Accessing Windows Folders";
LabelNotification.Text = "Please wait...";
foreach (string spath in SuspectedPath )
{
foreach (string vinlist in ListOfVirusToScanFor .ToArray ())
{
string scpath=spath + "\\" + vinlist ;
if (File .Exists (scpath )==true )
{
VirusPaths .Add (scpath );
}
}
}
}
}else
{
RegistryKey regkey =Registry.CurrentUser.OpenSubKey("Software\\Microsoft\\Windows\\CurrentVersion\\Policies\\System", true);
regkey.SetValue("DisableTaskMgr", 0, RegistryValueKind.DWord);
regkey.SetValue("DisableRegistryTools", 0, RegistryValueKind.DWord);
regkey.SetValue("NoFolderOptions", 0, RegistryValueKind.DWord);
regkey.SetValue("Hidden", 1, RegistryValueKind.DWord);
regkey.Close();
//open registry
regkey = Registry.CurrentUser.OpenSubKey("Software\\Microsoft\\Windows\\CurrentVersion\\Run", true);
foreach (string Startupfiles in regkey.GetValueNames())
{
foreach (string vinlist in ListOfVirusToScanFor.ToArray())
{
if (Startupfiles.Contains(vinlist) == true)
{
if (File.Exists(regkey.GetValue(Startupfiles).ToString()) == true)
{
VirusPaths.Add(regkey.GetValue(Startupfiles).ToString());
try
{
regkey.DeleteValue(regkey.GetValue(Startupfiles).ToString());
}
catch (Exception ex)
{
ErrorList.Add("Failed deleting '" + regkey.GetValue(Startupfiles).ToString() + "' because " + ex.Message);
}
}
}
else if (Startupfiles.StartsWith("rundll32.exe") == true)
{
VirusPaths.Add(regkey.GetValue(Startupfiles).ToString());
try
{
regkey.DeleteValue(regkey.GetValue(Startupfiles).ToString());
}
catch (Exception ex)
{
ErrorList.Add("Failed deleting '" + regkey.GetValue(Startupfiles).ToString() + "' because " + ex.Message);
}
}
else if (Startupfiles.Contains("ServiceDll") == true)
{
VirusPaths.Add(regkey.GetValue(Startupfiles).ToString());
try
{
regkey.DeleteValue(regkey.GetValue(Startupfiles).ToString());
}
catch (Exception ex)
{
ErrorList.Add("Failed deleting '" + regkey.GetValue(Startupfiles).ToString() + "' because " + ex.Message);
}
}
}
}
}
}
if (VirusPaths.Count > 0)
{
foreach (string suspectedFile in VirusPaths.ToArray())
{
Application.DoEvents();
//get path root
string getPathDrive = Path.GetPathRoot(suspectedFile);
try
{
//get drive name and label(volume)
string DriveName = new DriveInfo(getPathDrive).Name + new DriveInfo(getPathDrive).VolumeLabel;
}catch (Exception ex)
{
ErrorList.Add("failed to scan '" + getPathDrive + "' because " + ex.Message);
}
//search for shortcuts files in the checkeddrives path
foreach (string ShortcutFile in Directory.EnumerateFiles(getPathDrive, "*.lnk", SearchOption.TopDirectoryOnly))
{
Application.DoEvents();
try
{
LabelNotification.Text = "Deleting Shortcuts in " + getPathDrive;
//delete shortcut
File.Delete(ShortcutFile);
}
catch (Exception ex)
{
ErrorList.Add("Failed Deleting '" + ShortcutFile + "' because " + ex.Message);
}
}
LabelNotification.Text = "Getting folders in " + getPathDrive;
//get folders
foreach (string sfolder in Directory.EnumerateDirectories(getPathDrive))
{
Application.DoEvents();
DirectoryInfo foldertohide = new DirectoryInfo(sfolder);
LabelNotification.Text = "Unhidding folder(" + foldertohide.Name + ")";
try
{
//unhide folder
foldertohide.Attributes = FileAttributes.Normal;
}
catch (Exception ex)
{
ErrorList.Add("Failed unhidding folder '" + foldertohide.Name + "' because " + ex.Message);
}
}
//get files
foreach (string fileindrive in Directory.EnumerateFiles(getPathDrive, "*.*", SearchOption.TopDirectoryOnly))
{
Application.DoEvents();
FileInfo filetounhide = new FileInfo(fileindrive);
//check if filehave two extension
string checkextraext = fileindrive.Remove(fileindrive.Length - 4, 4);
if (File.Exists(checkextraext) == true)
{
try
{
File.Delete(fileindrive);
}
catch (Exception ex)
{
ErrorList.Add("Failed to delete '" + fileindrive + "' because " + ex.Message);
}
}
try
{
LabelNotification.Text = "Unhidding file (" + filetounhide.Name + ")";
//unhide folder
filetounhide.Attributes = FileAttributes.Normal;
}
catch (Exception ex)
{
ErrorList.Add("Failed unhidding file '" + filetounhide.Name + "' because " + ex.Message);
}
}
if (File.Exists(suspectedFile) == true)
{
try
{
File.Delete(suspectedFile);
}
catch (Exception ex)
{
ErrorList.Add("Failed deleting '" + suspectedFile + "' because " + ex.Message);
}
}
}
LabelNotification.Text = "Checking Registries.";
RegistryKey Regkey = Registry.CurrentUser.OpenSubKey("Software\\Microsoft\\Windows\\CurrentVersion\\Run", true);
foreach (string Regword in Regkey.GetValueNames())
{
string RegData = Regkey.GetValue(Regword).ToString();
foreach (string vbscript in VbList.ToArray())
{
//check if there is a .vbs file
if (RegData.Contains(vbscript) == true)
{
try
{//delete registry value
Regkey.DeleteValue(Regword);
}
catch (Exception ex)
{
ErrorList.Add("Failed accessing Registry because " + ex.Message);
}
}
}
}
WindowsIdentity Windentity = WindowsIdentity.GetCurrent ();
WindowsPrincipal wPrincipal = new WindowsPrincipal(Windentity);
if (wPrincipal.IsInRole(WindowsBuiltInRole.Administrator) == true)
{
RegistryKey Regkey2 = Registry.LocalMachine.OpenSubKey("Software\\Microsoft\\Windows\\CurrentVersion\\Run", true);
foreach (string Regword in Regkey2.GetValueNames())
{
string RegData = Regkey2.GetValue(Regword).ToString();
foreach (string vbscript in VbList.ToArray())
{
//check if there is a .vbs file
if (RegData.Contains(vbscript) == true)
{
try
{
//delete registry value
Regkey2.DeleteValue(Regword);
}
catch (Exception ex)
{
ErrorList.Add("Failed accessing Registry because " + ex.Message);
}
}
}
}
}
}
if (ErrorCount > 0)
{
//start windows explorer
Process.Start("Explorer.exe");
}
if (ErrorList.Count > 0)
{
buttonView.Visible = true;
}
LabelNotification.Text = "Done Scanning there were " + VirusPaths.Count + " files found.";
MessageBox.Show(LabelNotification.Text);
VirusPaths = new List<string>();
wsRunning = false;
ORunning = false;
ListView1.Enabled = buttonScan.Enabled = true;
buttonScan.Focus();
}
Int32 NoOfClick;
private void buttonView_Click(object sender, EventArgs e)
{
NoOfClick++;
if (NoOfClick == 1)
{
ListView1.Visible = buttonScan.Enabled = ButtonScanAs.Enabled = ComboBox1.Visible = LinkLabel1.Enabled = false;
ButtonSave.Visible = ListBox1.Visible = true;
buttonView.Text = "&Done";
foreach (string sd in ErrorList)
{
ListBox1.Items.Add(sd);
}
}
else if (NoOfClick ==2)
{
//clear listbox
ListBox1.Items.Clear();
ListView1.Visible = buttonScan.Enabled = ButtonScanAs.Enabled = ComboBox1.Visible = LinkLabel1.Enabled = true;
ButtonSave.Visible = ListBox1.Visible = false ;
//change buttonview text
buttonView.Text = "Vie&w Errors";
NoOfClick = 0;
}
}
private void ListView1_ItemChecked(object sender, ItemCheckedEventArgs e)
{
if (ListView1.CheckedItems.Count < 1)
{ComboBox1.SelectedIndex = 2;
//disable start button since there is nothing checked
buttonScan.Enabled = ButtonScanAs.Enabled = false;
}
else
{
ComboBox1.SelectedIndex = 1;
buttonScan.Enabled = true;
ButtonScanAs.Enabled = true;
}
}
private void ComboBox1_SelectedValueChanged(object sender, EventArgs e)
{
if (ComboBox1.SelectedIndex == 0)
{
//check all items in the listview
foreach (ListViewItem Litem in ListView1.Items)
{
Litem.Checked = true;
}
ComboBox1.SelectedIndex = 0;
}
else if (ComboBox1.SelectedIndex == 2)
{
//check all items in the listview
foreach (ListViewItem Litem in ListView1.Items)
{
Litem.Checked = false ;
}
ComboBox1.SelectedIndex = 2;
}
}
private void LinkLabel1_LinkClicked(object sender, LinkLabelLinkClickedEventArgs e)
{
ListView1.Items.Clear();
foreach (string USBorHdd in Environment.GetLogicalDrives())
{
//check if drive can be used
if (new DriveInfo(USBorHdd).IsReady == true)
{
if (new DriveInfo(USBorHdd).DriveType != DriveType.CDRom)
{
try
{
ListView1.Items.Add(USBorHdd + new DriveInfo(USBorHdd).VolumeLabel);
}
catch (Exception ex)
{
ErrorList.Add(ex.Message);
}
}
}
}
ComboBox1.SelectedIndex = 0;
}
private void ButtonSave_Click(object sender, EventArgs e)
{
SaveFileDialog sfd = new SaveFileDialog() { Filter = "Text Files|*.txt" };
if (sfd.ShowDialog() == DialogResult.OK)
{
Application.DoEvents();
LabelNotification.Text = "Please wait: Saving";
foreach (string sline in ListBox1.Items)
{
File.AppendAllText(sfd.FileName, sline + Environment .NewLine );
}
LabelNotification.Text = "Done Saving";
}
}
bool isElevated = false;
private void ButtonScanAs_Click(object sender, EventArgs e)
{
ProcessStartInfo ElevateProcess = new ProcessStartInfo();
ElevateProcess.FileName = Application.ExecutablePath;
ElevateProcess.WorkingDirectory = Environment.CurrentDirectory;
ElevateProcess.UseShellExecute = true;
ElevateProcess.Verb = "runas";
try
{
Process.Start(ElevateProcess);
}
catch (System.ComponentModel.Win32Exception )
{
return;
}
isElevated = true;
//close the current process
Application.Exit();
}
private void buttonClose_Click(object sender, EventArgs e)
{
this.Close();
}
private void CheckBoxForceScan_CheckedChanged(object sender, EventArgs e)
{
if (CheckBoxForceScan.Checked == true)
{
wsRunning = ORunning = true;
MessageBox.Show("Force Scan activated.");
buttonScan.Focus();
}
else
{
wsRunning = ORunning = false ;
MessageBox.Show("Force Scan is not activated.");
}
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
if (isElevated == true)
{
foreach (ListViewItem checkeditem in ListView1.CheckedItems)
{
try
{
File.AppendAllText(savecheckedfiles, checkeditem.Text + Environment .NewLine );
}
catch (Exception)
{
}
}
}
}
}
}