Notepad is a basic text editor that you can use to create simple documents. The most common use for Notepad is to view or edit text (.txt) files. This is a simple notepad clone written using the visual basic programming language. This can be useful for new or advance programmers who want to create a custom notepad.
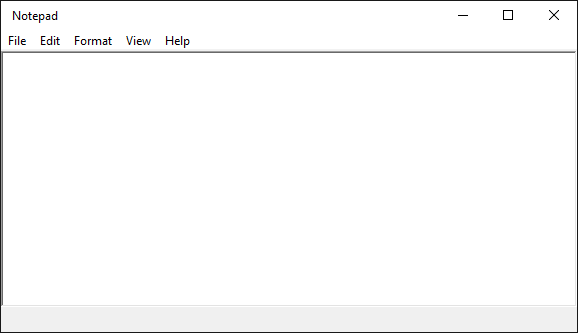
Code:
Public Class mainFrm
Inherits System.Windows.Forms.Form
Dim txtChanged As Boolean
Dim theStr As String
Private Sub txtPad_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles txtPad.TextChanged
txtChanged = True
linePanel.Text = "Ln: " & txtPad.Lines.Length
colPanel.Text = "Chr: " & txtPad.SelectionStart
End Sub
Private Sub mnuNew_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuNew.Click
Dim msg As String
If txtChanged = True Then
msg = MsgBox("Do you want to save your current changes?", MsgBoxStyle.YesNo, " Save Changes?")
End If
If msg = vbYes Then
Dim a As EventArgs
mnuSave_Click(0, a)
txtChanged = False
Else
txtRich.Clear()
txtChanged = False
End If
End Sub
Private Sub mnuPageSetup_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuPageSetup.Click
Dim dlgPageSetup As New PageSetupDialog()
End Sub
Private Sub mnuExit_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuExit.Click
Close()
End Sub
Private Sub mnuPrint_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuPrint.Click
Dim dlgPrint As New PrintDialog()
End Sub
Private Sub mnuOpen_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuOpen.Click
Dim openDlg As New OpenFileDialog()
Dim buff As String
openDlg.Filter = "Text Files (*.txt)|*.txt"
openDlg.ShowDialog()
FileOpen(1, openDlg.FileName, OpenMode.Input)
While Not EOF(1)
Input(1, buff)
txtRich.AppendText(buff)
End While
FileClose(1)
End Sub
Private Sub mnuSave_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuSave.Click
Dim saveDlg As New SaveFileDialog()
Dim buff As String
Static theFile As String
If System.IO.File.Exists(theFile) = False Then
saveDlg.Filter = "Text Files (*.txt)|*.txt"
saveDlg.ShowDialog()
If saveDlg.CheckFileExists = False Then Exit Sub
theFile = saveDlg.FileName
FileOpen(1, theFile, OpenMode.Output)
buff = txtRich.Text
Print(1, buff)
FileClose(1)
txtChanged = False
Else
FileOpen(1, theFile, OpenMode.Output)
buff = txtPad.Text
Print(1, buff)
FileClose(1)
txtChanged = False
End If
End Sub
Private Sub mnuSaveAs_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuSaveAs.Click
Dim saveDlg As New SaveFileDialog()
Dim theFile As String
Dim buff As String
saveDlg.Filter = "Text Files (*.txt)|*.txt"
saveDlg.ShowDialog()
If saveDlg.CheckFileExists = False Then Exit Sub
theFile = saveDlg.FileName
FileOpen(1, theFile, OpenMode.Output)
buff = txtRich.Text
Print(1, buff)
FileClose(1)
txtChanged = False
End Sub
Private Sub mnuUndo_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuUndo.Click
txtRich.Undo()
End Sub
Private Sub mnuTimeDate_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuTimeDate.Click
Dim theDate As Date
txtRich.AppendText(TimeOfDay & " " & Today)
End Sub
Private Sub mnuSelectAll_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuSelectAll.Click
txtRich.SelectAll()
End Sub
Private Sub mnuDel_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuDel.Click
txtRich.SelectedText = ""
End Sub
Private Sub mnuFind_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuFind.Click
theStr = InputBox("What do word do you want to search for?", " Find")
txtRich.Find(theStr)
End Sub
Private Sub mnuGoto_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuGoto.Click
Dim lineNum As Integer
Dim txtLineCount As Integer
txtLineCount = txtPad.Lines.Length
txtLineCount = CStr(txtLineCount)
lineNum = InputBox("Please enter the line number you want to goto.", " Goto Line Number", txtLineCount)
If lineNum > txtLineCount Then MsgBox("Line number is out of range!", MsgBoxStyle.Exclamation, " Out of range") : Exit Sub
lineNum = CInt(lineNum)
End Sub
Private Sub mnuCut_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuCut.Click
txtPad.Cut()
End Sub
Private Sub mnuCopy_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuCopy.Click
txtRich.Copy()
End Sub
Private Sub mnuPaste_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuPaste.Click
txtRich.Paste()
End Sub
Private Sub mnuWordwrap_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuWordwrap.Click
If mnuWordwrap.Checked = True Then
mnuWordwrap.Checked = False
txtRich.WordWrap = False
Else
mnuWordwrap.Checked = True
txtRich.WordWrap = True
End If
End Sub
Private Sub mnuFont_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuFont.Click
Dim fontDlg As New FontDialog()
fontDlg.ShowDialog()
txtRich.Font = fontDlg.Font
End Sub
Private Sub mnuStatusbar_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuStatusbar.Click
If mnuStatusbar.Checked = True Then
StatusBar1.Hide()
mnuStatusbar.Checked = False
Else
mnuStatusbar.Checked = True
StatusBar1.Show()
End If
End Sub
Private Sub mnuFindnext_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuFindnext.Click
Dim startStr As Integer
startStr = txtRich.SelectionStart
txtRich.Find(theStr, startStr, RichTextBoxFinds.WholeWord)
End Sub
Private Sub mnuReplace_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuReplace.Click
Dim strReplace As String
Dim strNew As String
If theStr = "" Then
theStr = InputBox("What word do you want to search for?", " Find")
If theStr = "" Then Exit Sub
End If
strNew = InputBox("What you do want to replace: " & theStr & " : with?", " Replace")
txtPad.SelectedText = strNew
End Sub
Private Sub txtRich_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles txtRich.TextChanged
txtChanged = True
linePanel.Text = "Ln: " & txtRich.Lines.Length
colPanel.Text = "Chr: " & txtRich.SelectionStart
End Sub
Private Sub MenuItem1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MenuItem1.Click
txtRich.Redo()
End Sub
End Class