write a program that will ask user to enter N Strings/Sentences.
The problem will display each string's information:
-Number of words
-Number of Spaces
-Reversed String (by Word)
Sample:
Number of string : 2
Enter String 1: I am Legend
Enter String 2: Hello Java
Result:
String 1 : I am Legend
Number of Words: 3
Number of spaces: 2
Reverse by word: I ma dnegeL
String 1 : Hello Java
Number of Words: 2
Number of spaces: 1
Reverse by word: olleH avaJ
Screen Shot:
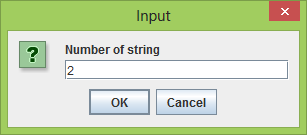
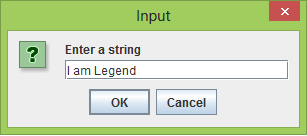
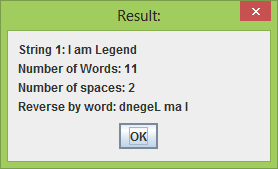
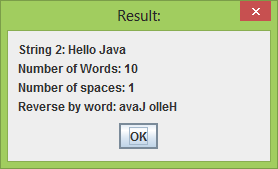
Code:
import javax.swing.JOptionPane;
public class reverse
{
public static void main(String[] args)
{
String []input_message = new String[100];;
String input_new = "";
int number_of_string;
String string_holder = "";
string_holder = JOptionPane.showInputDialog("Number of string");
number_of_string = Integer.parseInt(string_holder);
for(int i = 1; i <= number_of_string; i++)
{
input_message[i] = JOptionPane.showInputDialog("Enter a string ");
}
for(int i = 1; i <= number_of_string; i++)
{
int spaceCount = 0;
for (char c : input_message[i].toCharArray()) {
if (c == ' ') {
spaceCount++;
}
}
input_new = new StringBuffer(input_message[i]).reverse().toString();
JOptionPane.showMessageDialog(null,"String " + i + ": "+ input_message[i] + "\n" +"Number of Words: " + input_message[i].length() + "\n" +"Number of spaces: "+ spaceCount +"\n" + "Reverse by word: "+ input_new + " ", "Result:",JOptionPane.PLAIN_MESSAGE);
}
}
}