This is a simple program that Enable/Disable USB ports on a PC. A USB port is a standard cable connection interface for personal computers and consumer electronics devices. USB stands for Universal Serial Bus, an industry standard for short-distance digital data communications.
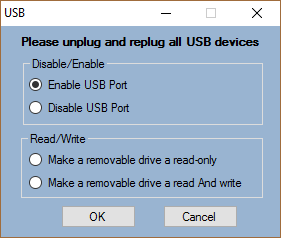
Code:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Runtime.InteropServices;
using Microsoft.Win32;
using System.Threading;
using System.Windows.Forms;
namespace USBEnableDisable
{
public partial class frmMain : Form
{
public frmMain()
{
InitializeComponent();
}
RegistryKey Regkey, RegKey2;
Int32 rValue,rsvalue,Gvalue,tvalue;
string Regpath = "System\\CurrentControlSet\\Services\\USBSTOR";
string ReadAndWriteRegPath2 = "System\\CurrentControlSet\\Control";
string ReadAndWriteRegPath = "System\\CurrentControlSet\\Control\\StorageDevicePolicies";
private void RadioButtondisable_CheckedChanged(object sender, EventArgs e)
{
GroupBox2.Enabled = false;
rValue = 4;
}
private void RadioButtonenable_CheckedChanged(object sender, EventArgs e)
{
GroupBox2.Enabled = true;
rValue = 3;
}
private void RadioButtonreadonly_CheckedChanged(object sender, EventArgs e)
{
rsvalue = 1;
}
private void btnOK_Click(object sender, EventArgs e)
{
Regkey = Registry.LocalMachine.OpenSubKey(Regpath, true);
Regkey.SetValue("Start", rValue);
if (GroupBox2.Enabled == true)
{
RegKey2 = Registry.LocalMachine.OpenSubKey(ReadAndWriteRegPath2, true);
RegKey2.CreateSubKey("StorageDevicePolicies");
RegKey2 = Registry.LocalMachine.OpenSubKey(ReadAndWriteRegPath, true);
RegKey2.SetValue("WriteProtect", rsvalue);
}
if ((rValue == 3) && (rsvalue == 1))
{
MessageBox.Show("USB Port were enable and Read only is enabled");
}
else if ((rValue == 3) && (rsvalue == 0))
{
MessageBox.Show("USB Port were enable and Read and write is enabled");
}
else
{
MessageBox.Show("USB Port were disable");
}
}
private void frmMain_Load(object sender, EventArgs e)
{
isAdmin = IsUserAnAdmin();
if (isAdmin == false)
{
MessageBox.Show("You don't have proper privileges level to make changes, administrators privileges are required", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
Close();
}
else
{
Regkey = Registry.LocalMachine.OpenSubKey(Regpath, true);
Gvalue = Convert.ToInt32(Regkey.GetValue("Start"));
//check the current state of the usb/whether is enabled or disabled
if (Gvalue == 3)
{
rdoEnable.Checked = true;
}
else if (Gvalue == 4)
{
rdoDisable.Checked = true;
}
RegKey2 = Registry.LocalMachine.OpenSubKey(ReadAndWriteRegPath, true);
try
{
tvalue = Convert.ToInt32(RegKey2.GetValue("WriteProtect"));
if (tvalue == 1)
{
RadioButtonreadonly.Checked = true;
}
else if (tvalue == 0)
{
RadioButtonreadwrite.Checked = true;
}
}
catch (NullReferenceException) { }
}
}
private void btnCancel_Click(object sender, EventArgs e)
{
Close();
}
private void RadioButtonreadwrite_CheckedChanged(object sender, EventArgs e)
{
rsvalue = 0;
}
bool isAdmin;
[DllImport ("shell32")]
static extern bool IsUserAnAdmin();
}
}