This program is used to format a USB Drive or a hard drive.
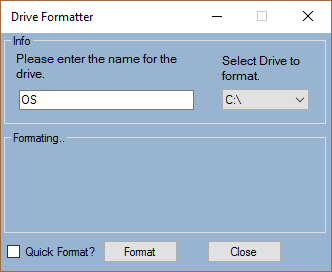
Code:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using Microsoft.VisualBasic;
using Microsoft.VisualBasic.ApplicationServices;
using System.Linq;
using System.IO;
using System.Diagnostics;
using System.Text;
using System.Windows.Forms;
namespace Drive_Formatter
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
string request,strName;
Process MyProcess = new Process();
private void button1_Click(object sender, EventArgs e)
{
DriveInfo SelectedDrive = new DriveInfo(comboBoxDrives.Text);
if (MessageBox.Show("Do you really want to format?", "Info", MessageBoxButtons.YesNo) == DialogResult.Yes)
{
if (SelectedDrive.DriveType == DriveType.Removable)
{
//check if quick format checkbox is checked
if (checkBoxQuickformat.Checked == false)
{
strName = textBoxVolumeLabel.Text.Trim();
request = "Format /v:" + strName + " /x /backup " + SelectedDrive.Name.Replace("\\", " ");
MyProcess.StartInfo.RedirectStandardOutput = true;
MyProcess.StartInfo.RedirectStandardError = true;
MyProcess.StartInfo.CreateNoWindow = true;
MyProcess.StartInfo.UseShellExecute = false;
//this try statement is here to avoid the application to crash,
//when the is no enough space, read-only location
try
{
File.WriteAllText("batman.bat", request + Environment.NewLine);
}
catch (IOException m)
{
MessageBox.Show(m.Message.ToString());
buttonFormat.Enabled = true;
return;
}
MyProcess.StartInfo.FileName = "batman.bat";
//START the process
MyProcess.Start();
timer1.Enabled = true;
}
else
{
strName = textBoxVolumeLabel.Text.Trim();
//note the below line can also work on Limited/Standard user
request = "Format /v:" + strName + " /x /Q /backup " + SelectedDrive.Name.Replace("\\", " ");
MyProcess.StartInfo.RedirectStandardOutput = true;
MyProcess.StartInfo.RedirectStandardError = true;
MyProcess.StartInfo.CreateNoWindow = true;
MyProcess.StartInfo.UseShellExecute = false;
//this try statement is here to avoid the application to crash,
//when the is no enough space, read-only location
try
{
File.WriteAllText("batman.bat", request + Environment.NewLine);
}
catch (IOException m)
{
MessageBox.Show(m.Message.ToString());
buttonFormat.Enabled = true;
return;
}
MyProcess.StartInfo.FileName = "batman.bat";
//START the process
MyProcess.Start();
timer1.Enabled = true;
}
}
else if (SelectedDrive.DriveType == DriveType.Fixed)
{
if (checkBoxQuickformat.Checked == false)
{
MyProcess.StartInfo.RedirectStandardOutput = true;
MyProcess.StartInfo.RedirectStandardError = true;
MyProcess.StartInfo.CreateNoWindow = true;
MyProcess.StartInfo.UseShellExecute = false;
try
{
File.WriteAllText("batman.bat", "Format /x /v:" + textBoxVolumeLabel.Text + " " + comboBoxDrives.SelectedItem.ToString());
}
catch (IOException m)
{
MessageBox.Show(m.Message.ToString());
buttonFormat.Enabled = true;
return;
}
MyProcess.StartInfo.FileName = "batman.bat";
//START the process
MyProcess.Start();
timer1.Enabled = true;
}
else
{
MyProcess.StartInfo.RedirectStandardOutput = true;
MyProcess.StartInfo.RedirectStandardError = true;
MyProcess.StartInfo.CreateNoWindow = true;
MyProcess.StartInfo.UseShellExecute = false;
try
{
File.WriteAllText("batman.bat", "Format /q /x /v:" + textBoxVolumeLabel.Text + " " + comboBoxDrives.SelectedItem.ToString());
}
catch (IOException m)
{
MessageBox.Show(m.Message.ToString());
buttonFormat.Enabled = true;
return;
}
MyProcess.StartInfo.FileName = "batman.bat";
//START the process
MyProcess.Start();
timer1.Enabled = true;
}
}
}
}
bool isFormating=false ;
private void timer1_Tick(object sender, EventArgs e)
{
if (labelProgress.Text.Contains("Volume Serial") == false)
{
//check if format was aborted
if (labelProgress.Text.Contains("Format aborted.") == false)
{
labelInfo.Text = "Please wait, there formater is busy...";
//get the current progress
labelProgress.Text = MyProcess.StandardOutput.ReadLine().ToString();
//disable the format button
buttonFormat.Enabled = false;
isFormating = true;
}
else {
timer1.Enabled = false;
labelInfo.Text = "Format aborted.";
MessageBox.Show(labelInfo.Text, "Error");
labelProgress.Text = string.Empty;
isFormating = false;
buttonFormat.Enabled = true;
}
if (labelProgress.Text.Contains("Enter current volume label") == true )
{
StreamWriter stremwriter ;
stremwriter = MyProcess.StandardInput;
stremwriter.Write(FixedHardDriveName);
stremwriter.Close();
}
}
else
{
timer1.Enabled = false;
labelInfo.Text = "Done Formating";
labelProgress.Text = string.Empty;
MessageBox.Show(labelInfo.Text);
isFormating = false;
buttonFormat.Enabled = true;
}
}
private void textBoxVolumeLabel_KeyPress(object sender, KeyPressEventArgs e)
{
//allow only alphabets to be entered in the textbox
if (((e.KeyChar < 'a') || (e.KeyChar > 'z')) && ((e.KeyChar < 'A') || (e.KeyChar > 'Z' )))
{
e.Handled = true;
}
}
Microsoft.VisualBasic.ApplicationServices.User Myuser;
private void Form1_Load(object sender, EventArgs e)
{
Myuser = new User();
if (Myuser.IsInRole("Administrators") != true)
{
//get all attached removable drives
foreach (string AttachedDrive in Environment.GetLogicalDrives())
{
DriveInfo CompDrive = new DriveInfo(AttachedDrive);
if ((CompDrive.Name != "A:\\") && (CompDrive.Name != "B:\\"))
{
//allow only removable and fixed drives to be added in the combobox
if ((CompDrive.DriveType == DriveType.Removable) || (CompDrive.DriveType == DriveType.Fixed))
{
//add drive name into the combobox
comboBoxDrives.Items.Add(CompDrive.Name);
}
}
}
//select the first item on the combobox
comboBoxDrives.SelectedIndex = 0;
}
else
{
MessageBox.Show("Administrators prevelegies required.");
this.Close();
}
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
if (isFormating == true)
{
e.Cancel = false;
}
else
{
if (File.Exists("batman.bat") == true)
{
//delete the batman.bat file
File.Delete("batman.bat");
}
}
//check if the application is closed by a taskmanager
if (e.CloseReason == CloseReason.TaskManagerClosing)
{
if (File.Exists("batman.bat") == true)
{
//delete the batman.bat file
File.Delete("batman.bat");
}
}
}
private void buttonClose_Click(object sender, EventArgs e)
{
if (isFormating == false)
{
if (File.Exists("batman.bat") == true)
{
//delete the batman.bat file
File.Delete("batman.bat");
this.Close();
}
}
}
string FixedHardDriveName;
private void comboBoxDrives_SelectedValueChanged(object sender, EventArgs e)
{
DriveInfo SelectedDrive = new DriveInfo(comboBoxDrives.Text);
if (SelectedDrive.IsReady == true )
{
if (SelectedDrive.VolumeLabel == String.Empty )
{
textBoxVolumeLabel.Text = "Removable Disc";
FixedHardDriveName = SelectedDrive.VolumeLabel;}
else{
FixedHardDriveName = SelectedDrive.VolumeLabel;
textBoxVolumeLabel.Text = SelectedDrive.VolumeLabel;
}
}
}
}
}