Have you ever wanted to create a custom web browser? I will show you how to create a very simple custom web browser using WPF and C# with very little code. A web browser is a software application for retrieving, presenting and traversing information resources on the World Wide Web. The major web browsers are Google Chrome, Mozilla Firefox, Internet Explorer, Opera, and Safari. Feel free to experiment with the code and customize it as much as you want. This very simple browser only as a back, address bar, forward, go and refresh button. The Back and forward buttons go back to the previous resource and forward respectively. The refresh or reload button reload the current resource. The address bar input the Uniform Resource Identifier (URI) of the desired resource and display it.
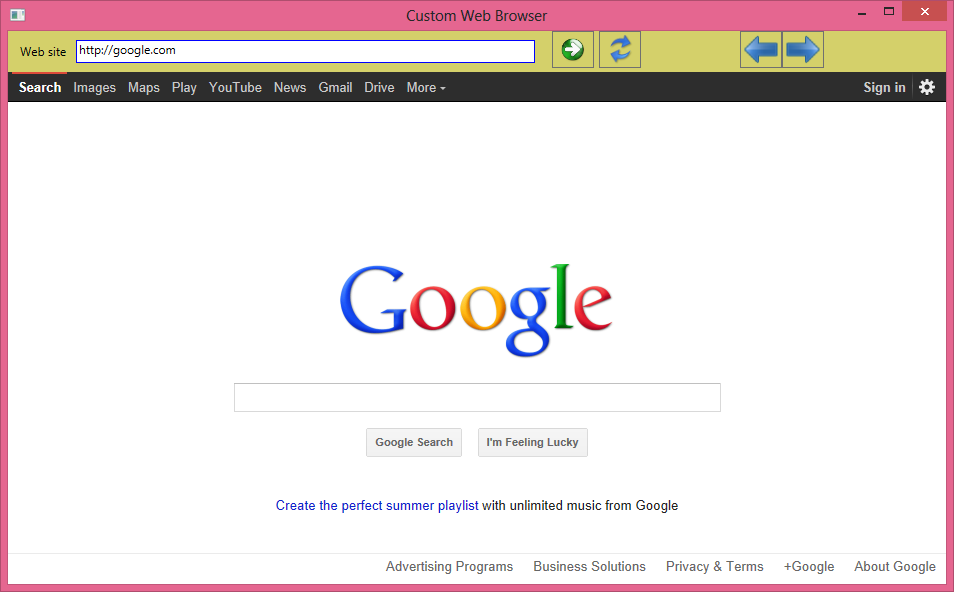
XAML Code:
<Window x:Class="WebBrowserApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Custom Web Browser" Height="592" Width="954"
WindowStartupLocation="CenterScreen" >
<Grid Background="#FFD4D06A">
<WebBrowser Name="webBrowser1" Margin="0,41,0,0" />
<TextBox Name="webSiteAdr" Height="23" HorizontalAlignment="Left"
Margin="68,9,0,0" VerticalAlignment="Top" Width="459"
Text="http://code.msdn.microsoft.com/" BorderBrush="Blue" />
<TextBlock Height="23" HorizontalAlignment="Left" Margin="12,12,0,0"
Name="textBlock1" Text="Web site" VerticalAlignment="Top" Width="50" />
<Button Height="37" HorizontalAlignment="Left" Margin="544,0,0,0" Name="button1"
Background="Transparent" VerticalAlignment="Top" Width="42" Click="goButtonClick">
<Image Source="Images/forward_button.png" Width="37" />
</Button>
<Button Height="37" HorizontalAlignment="Left" Margin="591,0,0,0" Name="button2"
Background="Transparent" VerticalAlignment="Top" Width="42" Click="goButtonClick">
<Image Source="Images/refresh.png" Height="29" />
</Button>
<Button Height="37" HorizontalAlignment="Left" Margin="732,0,0,0" Name="button3"
Background="Transparent" VerticalAlignment="Top" Width="42" Click="backButtonClick">
<Image Source="Images/backward.png" Width="37" />
</Button>
<Button Height="37" HorizontalAlignment="Left" Margin="774,0,0,0" Name="button4"
Background="Transparent" VerticalAlignment="Top" Width="42" Click="forwardButtonClick">
<Image Source="Images/forward.png" Width="37" />
</Button>
</Grid>
</Window>
C# Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace WebBrowserApp
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void goButtonClick(object sender, RoutedEventArgs e)
{
webBrowser1.Navigate(webSiteAdr.Text);
}
private void refreshButtonClick(object sender, RoutedEventArgs e)
{
webBrowser1.Refresh();
}
private void backButtonClick(object sender, RoutedEventArgs e)
{
webBrowser1.GoBack();
}
private void forwardButtonClick(object sender, RoutedEventArgs e)
{
webBrowser1.GoForward();
}
}
}