Want to allow users to crop images on your website? Or maybe you just simple want to know how to do this. Look no further you are in good hands. I will be using a JQuery Plugin called JCrop to do this. Jcrop is the quick and easy way to add image cropping functionality to your web application. It combines the ease-of-use of a typical jQuery plugin with a powerful cross-platform DHTML cropping engine that is faithful to familiar desktop graphics applications. The code will be provided below. There is also a download link for the project. Enjoy!
JCrop Currently have the following features:
Attaches unobtrusively to images or block objects
Supports aspect ratio locking
Supports minSize/maxSize setting
Callbacks for selection done, or while moving
Keyboard support for nudging selection
API features to create interactivity, including animation
Support for CSS styling, now uses LESS
Touch support for iOS, Android, etc
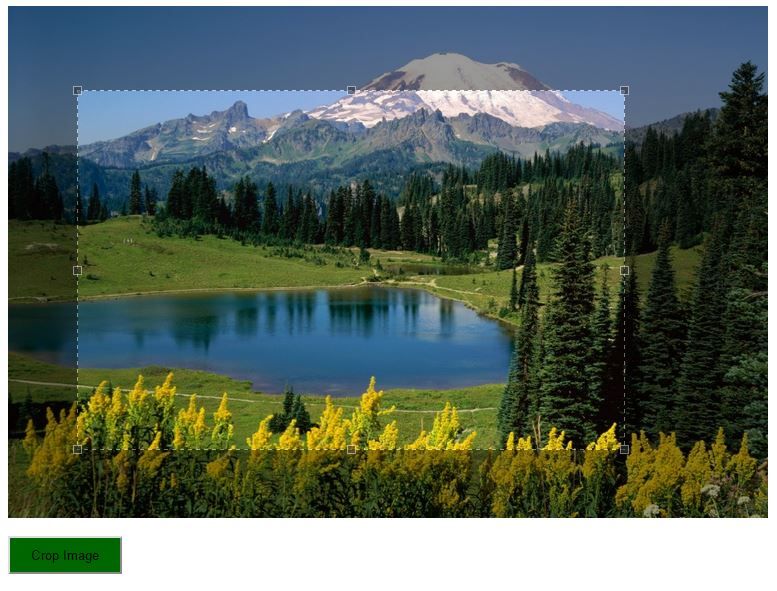
aspx:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="UploadAndCrop.aspx.cs" Inherits="UploadAndCrop" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title></title>
<link href="css/jquery.Jcrop.css" rel="stylesheet" type="text/css" />
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.3/jquery.min.js"></script>
<script type="text/javascript" src="js/jquery.Jcrop.js"></script>
<script type="text/javascript" src="js/jquery.Jcrop.min.js"></script>
<script type="text/javascript">
jQuery(document).ready(function() {
jQuery('#imgCrop').Jcrop({
onSelect: storeCoords
});
});
function storeCoords(c) {
jQuery('#X').val(c.x);
jQuery('#Y').val(c.y);
jQuery('#W').val(c.w);
jQuery('#H').val(c.h);
};
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Panel ID="pnlCrop" runat="server" >
<asp:Image ID="imgCrop" runat="server" ImageUrl="~/images/nature.jpg" Height="512px" Width="760px" />
<br />
<asp:HiddenField ID="X" runat="server" />
<asp:HiddenField ID="Y" runat="server" />
<asp:HiddenField ID="W" runat="server" />
<asp:HiddenField ID="H" runat="server" />
<asp:Button ID="btnCrop" runat="server" Text="Crop Image" OnClick="btnCrop_Click" BackColor="#006600" Height="38px" Width="114px" />
</asp:Panel>
</div>
</form>
</body>
</html>
cs:
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.IO;
using SD = System.Drawing;
using System.Drawing.Imaging;
using System.Drawing.Drawing2D;
public partial class UploadAndCrop : System.Web.UI.Page
{
String path = HttpContext.Current.Request.PhysicalApplicationPath + "images\\";
String fileLocation = HttpContext.Current.Server.MapPath("~/images/nature.jpg");
protected void btnCrop_Click(object sender, EventArgs e)
{
int w = Convert.ToInt32(W.Value);
int h = Convert.ToInt32(H.Value);
int x = Convert.ToInt32(X.Value);
int y = Convert.ToInt32(Y.Value);
byte[] CropImage = Crop(fileLocation, w, h, x, y);
using (MemoryStream ms = new MemoryStream(CropImage, 0, CropImage.Length))
{
ms.Write(CropImage, 0, CropImage.Length);
using (SD.Image CroppedImage = SD.Image.FromStream(ms, true))
{
string SaveTo = path + "Newnature.jpg";
CroppedImage.Save(SaveTo, CroppedImage.RawFormat);
imgCrop.ImageUrl = "~/images/Newnature.jpg";
}
}
}
static byte[] Crop(string Img, int Width, int Height, int X, int Y)
{
try
{
using (SD.Image OriginalImage = SD.Image.FromFile(Img))
{
using (SD.Bitmap bmp = new SD.Bitmap(Width, Height))
{
bmp.SetResolution(OriginalImage.HorizontalResolution, OriginalImage.VerticalResolution);
using (SD.Graphics Graphic = SD.Graphics.FromImage(bmp))
{
Graphic.SmoothingMode = SmoothingMode.AntiAlias;
Graphic.InterpolationMode = InterpolationMode.HighQualityBicubic;
Graphic.PixelOffsetMode = PixelOffsetMode.HighQuality;
Graphic.DrawImage(OriginalImage, new SD.Rectangle(0, 0, Width, Height), X, Y, Width, Height, SD.GraphicsUnit.Pixel);
MemoryStream ms = new MemoryStream();
bmp.Save(ms, OriginalImage.RawFormat);
return ms.GetBuffer();
}
}
}
}
catch (Exception Ex)
{
throw (Ex);
}
}
}
Download Link: https://drive.google.com/file/d/0B6Ukp4glmI9NQ05DM3BSWkdMTzg/view