Write a program that asks for the names of three runners and the time it took each of them to finish the race. The program should display who came in first, second, and third place.
Input Validation: Only accept positive numbers for the times.
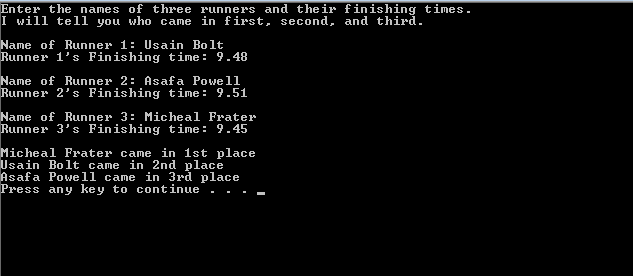
Code:
#include <iostream>
#include <string>
#include <cstdlib>
using namespace std;
void Input_Validation(float runner_time);
int main(int argc, char *argv[])
{
float max=0,min=0;
string runner1;
float runner1_time;
string runner2;
float runner2_time;
string runner3;
float runner3_time;
cout<<"Enter the names of three runners and their finishing times."<<endl;
cout<<"I will tell you who came in first, second, and third."<<endl<<endl;
cout<<"Name of Runner 1: ";
getline(cin,runner1);
cout<<"Runner 1's Finishing time: ";
cin>>runner1_time;
Input_Validation(runner1_time);
cin.ignore(1);
cout<<endl<<"Name of Runner 2: ";
getline(cin,runner2);
cout<<"Runner 2's Finishing time: ";
cin>>runner2_time;
Input_Validation(runner2_time);
cin.ignore(1);
cout<<endl<<"Name of Runner 3: ";
getline(cin,runner3);
cout<<"Runner 3's Finishing time: ";
cin>>runner3_time;
Input_Validation(runner3_time);
cin.ignore(1);
cout<<endl;
if(runner1_time>runner2_time)
{
max=runner1_time; min=runner2_time;
}
else
{
max=runner2_time; min=runner1_time;
}
if(runner3_time>max)
max=runner3_time;
else
min=runner3_time;
if(runner1_time == min)
{
cout<<runner1<<" came in 1st place"<<endl;
}
else if(runner2_time == min)
{
cout<<runner2<<" came in 1st place"<<endl;
}
else if(runner3_time == min)
{
cout<<runner3<<" came in 1st place"<<endl;
}
if(runner1_time != max || runner1_time != min)
{
cout<<runner1<<" came in 2nd place"<<endl;
}
else if(runner2_time != max || runner2_time != min)
{
cout<<runner2<<" came in 2nd place"<<endl;
}
else if(runner3_time != max || runner3_time != min)
{
cout<<runner3<<" came in 2nd place"<<endl;
}
if(runner1_time == max)
{
cout<<runner1<<" came in 3rd place"<<endl;
}
else if(runner2_time == max)
{
cout<<runner2<<" came in 3rd place"<<endl;
}
else if(runner3_time == max)
{
cout<<runner3<<" came in 3rd place"<<endl;
}
return 0;
}
void Input_Validation(float runner_time)
{
if(runner_time < 0)
{
cout<<"Positive number only"<<endl;
system("pause");
exit(0);
}
}