This program will change the time and date on a PC.
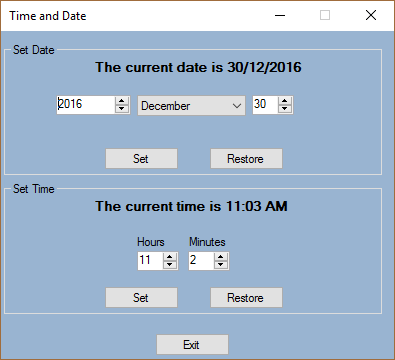
Code:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Diagnostics;
using System.IO;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace TimeAndDateChanger
{
public partial class frmTimeandDate : Form
{
public frmTimeandDate()
{
InitializeComponent();
}
Decimal Hour, Minutes;
decimal GetYear,getmonth,getdate;
Int32 month;
private void buttonsettime_Click(object sender, EventArgs e)
{
StreamWriter streamtext;
//write time in the bat file
File.WriteAllText("man.bat", "time");
Process myprocess = new Process();
myprocess.StartInfo.CreateNoWindow = true;
myprocess.StartInfo.RedirectStandardInput = true;
myprocess.StartInfo.RedirectStandardOutput = true;
myprocess.StartInfo.UseShellExecute = false;
myprocess.StartInfo.FileName = "man.bat";
//start the bat file
myprocess.Start();
streamtext = myprocess.StandardInput;
//write the numeric values into the streamwriter
streamtext.Write(numericUpDownhour.Value + ":" + numericUpDownminutes.Value );
streamtext.Flush();
streamtext.Close();
}
private void buttonRestoretime_Click(object sender, EventArgs e)
{
StreamWriter streamtext;
File.WriteAllText("man.bat", "time");
//create a new process
Process myprocess = new Process();
//create nowindow of the batch file
myprocess.StartInfo.CreateNoWindow = true;
myprocess.StartInfo.RedirectStandardInput = true;
myprocess.StartInfo.RedirectStandardOutput = true;
myprocess.StartInfo.UseShellExecute = false;
myprocess.StartInfo.FileName = "man.bat";
myprocess.Start();
streamtext = myprocess.StandardInput;
streamtext.Write(Hour + ":" + Minutes );
//save the changes made
streamtext.Flush();
streamtext.Close();
}
private void buttonExit_Click(object sender, EventArgs e)
{
//delete the bat file
File.Delete("man.bat");
this.Close();
}
private void frmTimeandDate_Load(object sender, EventArgs e)
{
getmonth = comboBoxmonths.SelectedIndex = DateTime.Now.Month - 1;
Hour = numericUpDownhour.Value = DateTime.Now.Hour;
getdate = numericUpDowndate.Value = Convert.ToInt32(DateTime.Now.Date.ToString().Substring(0, 2).Trim());
Minutes = numericUpDownminutes.Value = DateTime.Now.Minute;
GetYear = numericUpDownyear.Value = DateTime.Now.Year;
tmTimer.Enabled = true;
}
private void btnSetDate_Click(object sender, EventArgs e)
{
StreamWriter streamtext;
File.WriteAllText("man.bat", "date");
//create a new process
Process myprocess = new Process();
//create nowindow of the batch file
myprocess.StartInfo.CreateNoWindow = true;
myprocess.StartInfo.RedirectStandardInput = true;
myprocess.StartInfo.RedirectStandardOutput = true;
myprocess.StartInfo.UseShellExecute = false;
myprocess.StartInfo.FileName = "man.bat";
myprocess.Start();
streamtext = myprocess.StandardInput;
streamtext.Write(numericUpDowndate.Value + "-" + month + "-" + numericUpDownyear.Value);
//save the changes made
streamtext.Flush();
streamtext.Close();
}
private void tmTimer_Tick(object sender, EventArgs e)
{
//display the current time
labelcurrenttime.Text = "The current time is " + DateTime.Now.ToShortTimeString();
//display the current date
labelcurrentdate.Text = "The current date is " + DateTime.Now.ToShortDateString();
}
private void comboBoxmonths_SelectedIndexChanged(object sender, EventArgs e)
{
try
{
month = comboBoxmonths.SelectedIndex + 1;
numericUpDowndate.Maximum = Application.CurrentCulture.Calendar.GetDaysInMonth((Int32)numericUpDownyear.Value, comboBoxmonths.SelectedIndex + 1);
}catch (ArgumentOutOfRangeException)
{
numericUpDowndate.Maximum = Application.CurrentCulture.Calendar.GetDaysInMonth(DateTime .Now .Year , comboBoxmonths.SelectedIndex + 1);
}
}
Int32 dmonth;
private void buttonRestoredate_Click(object sender, EventArgs e)
{
StreamWriter streamtext;
File.WriteAllText("man.bat", "date");
//create a new process
Process myprocess = new Process();
//create nowindow of the batch file
myprocess.StartInfo.CreateNoWindow = true;
myprocess.StartInfo.RedirectStandardInput = true;
myprocess.StartInfo.RedirectStandardOutput = true;
myprocess.StartInfo.UseShellExecute = false;
myprocess.StartInfo.FileName = "man.bat";
myprocess.Start();
streamtext = myprocess.StandardInput;
dmonth =Convert .ToInt32 ( getmonth + 1);
//set the time
streamtext.Write(getdate + "-" + dmonth + "-" + GetYear );
//save the changes made
streamtext.Flush();
streamtext.Close();
numericUpDownyear.Value = GetYear;
numericUpDowndate.Value = getdate;
comboBoxmonths.SelectedIndex =(Int32 ) getmonth;
}
}
}